用c++编写程序从键盘输入5个学生的信息,包括学生学号、姓名、性别、出生年月及成绩,用结构体数组保存学生的信息,要求用函数实现结构体数组的输入输出及求成绩最高学生的序号。
时间: 2024-01-06 10:07:04 浏览: 59
好的,下面是用 C++ 编写的程序,它可以从键盘输入 5 个学生的信息,包括学生学号、姓名、性别、出生年月及成绩,用结构体数组保存学生的信息,还可以用函数实现结构体数组的输入输出及求成绩最高学生的序号:
```c++
#include <iostream>
#include <string>
using namespace std;
struct Student {
int id;
string name;
char gender;
string birthday;
float score;
};
void input(Student students[], int n) {
for (int i = 0; i < n; i++) {
cout << "请输入第 " << i + 1 << " 个学生的信息:" << endl;
cout << "学号:";
cin >> students[i].id;
cout << "姓名:";
cin >> students[i].name;
cout << "性别:";
cin >> students[i].gender;
cout << "出生年月:";
cin >> students[i].birthday;
cout << "成绩:";
cin >> students[i].score;
cout << endl;
}
}
void output(Student students[], int n) {
cout << "学生信息如下:" << endl;
for (int i = 0; i < n; i++) {
cout << "学号:" << students[i].id << endl;
cout << "姓名:" << students[i].name << endl;
cout << "性别:" << students[i].gender << endl;
cout << "出生年月:" << students[i].birthday << endl;
cout << "成绩:" << students[i].score << endl;
cout << endl;
}
}
int getMaxScoreIndex(Student students[], int n) {
int maxIndex = 0;
for (int i = 1; i < n; i++) {
if (students[i].score > students[maxIndex].score) {
maxIndex = i;
}
}
return maxIndex;
}
int main() {
Student students[5];
input(students, 5);
output(students, 5);
int maxIndex = getMaxScoreIndex(students, 5);
cout << "成绩最高的学生是:" << students[maxIndex].name << endl;
return 0;
}
```
在这个程序中,我们首先定义了一个结构体 `Student`,这个结构体包括了学生学号、姓名、性别、出生年月和成绩这 5 个成员变量。
然后,我们定义了 3 个函数,分别是:
- `input()`:用于从键盘输入 5 个学生的信息,包括学生学号、姓名、性别、出生年月和成绩,把这些信息保存到一个结构体数组中。
- `output()`:用于把结构体数组中的学生信息输出到屏幕上。
- `getMaxScoreIndex()`:用于求出结构体数组中成绩最高的学生的序号。
最后,我们在 `main()` 函数中调用这 3 个函数,完成了从键盘输入 5 个学生的信息,包括学生学号、姓名、性别、出生年月及成绩,用结构体数组保存学生的信息,要求用函数实现结构体数组的输入输出及求成绩最高学生的序号这个需求。
注意,这个程序使用了 `string` 类型来保存学生的姓名和出生年月,这个类型需要 `#include <string>`。
相关推荐
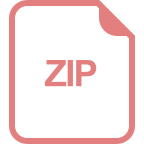
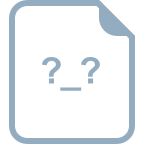
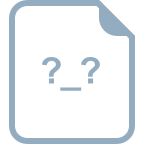














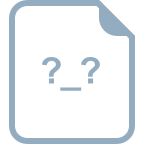