帮我用python写一个贪吃蛇
时间: 2023-06-15 07:04:23 浏览: 50
好的,以下是一个简单的贪吃蛇游戏代码示例:
```python
import pygame
import random
# 全局变量
SCREEN_WIDTH = 600
SCREEN_HEIGHT = 600
BLOCK_SIZE = 20
# 蛇类
class Snake:
def __init__(self):
self.body = [(SCREEN_WIDTH/2, SCREEN_HEIGHT/2)]
self.direction = 'right'
self.score = 0
self.is_dead = False
# 移动蛇
def move(self):
head = self.body[0]
if self.direction == 'right':
new_head = (head[0] + BLOCK_SIZE, head[1])
elif self.direction == 'left':
new_head = (head[0] - BLOCK_SIZE, head[1])
elif self.direction == 'up':
new_head = (head[0], head[1] - BLOCK_SIZE)
elif self.direction == 'down':
new_head = (head[0], head[1] + BLOCK_SIZE)
self.body.insert(0, new_head)
self.body.pop()
# 改变方向
def change_direction(self, direction):
if direction == 'right' and self.direction != 'left':
self.direction = 'right'
elif direction == 'left' and self.direction != 'right':
self.direction = 'left'
elif direction == 'up' and self.direction != 'down':
self.direction = 'up'
elif direction == 'down' and self.direction != 'up':
self.direction = 'down'
# 吃食物
def eat_food(self, food):
if self.body[0] == food.position:
self.body.insert(0, food.position)
self.score += 1
return True
return False
# 检测是否撞墙或自身
def check_collision(self):
head = self.body[0]
if head[0] < 0 or head[0] >= SCREEN_WIDTH or head[1] < 0 or head[1] >= SCREEN_HEIGHT:
self.is_dead = True
for block in self.body[1:]:
if head == block:
self.is_dead = True
# 食物类
class Food:
def __init__(self):
self.position = self.generate_position()
# 生成随机位置
def generate_position(self):
x = random.randint(0, SCREEN_WIDTH/BLOCK_SIZE - 1) * BLOCK_SIZE
y = random.randint(0, SCREEN_HEIGHT/BLOCK_SIZE - 1) * BLOCK_SIZE
return (x, y)
# 重新生成位置
def regenerate(self):
self.position = self.generate_position()
# 主函数
def main():
# 初始化
pygame.init()
pygame.display.set_caption('贪吃蛇')
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
clock = pygame.time.Clock()
font = pygame.font.SysFont('Arial', 30)
snake = Snake()
food = Food()
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
return
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_RIGHT:
snake.change_direction('right')
elif event.key == pygame.K_LEFT:
snake.change_direction('left')
elif event.key == pygame.K_UP:
snake.change_direction('up')
elif event.key == pygame.K_DOWN:
snake.change_direction('down')
# 移动蛇
snake.move()
# 检测是否吃到食物
if snake.eat_food(food):
food.regenerate()
# 检测是否撞墙或自身
snake.check_collision()
# 绘制画面
screen.fill((255, 255, 255))
for block in snake.body:
pygame.draw.rect(screen, (0, 255, 0), (block[0], block[1], BLOCK_SIZE, BLOCK_SIZE))
pygame.draw.rect(screen, (255, 0, 0), (food.position[0], food.position[1], BLOCK_SIZE, BLOCK_SIZE))
score_text = font.render('Score: ' + str(snake.score), True, (0, 0, 0))
screen.blit(score_text, (10, 10))
if snake.is_dead:
game_over_text = font.render('Game Over', True, (255, 0, 0))
screen.blit(game_over_text, (SCREEN_WIDTH/2 - 80, SCREEN_HEIGHT/2))
pygame.display.update()
clock.tick(10)
if __name__ == '__main__':
main()
```
你可以将代码保存为一个名为 `snake.py` 的文件,然后在命令行中运行 `python snake.py` 来启动游戏。
相关推荐
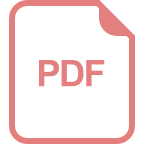
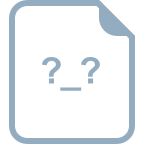
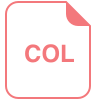

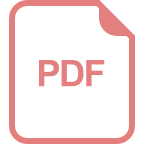
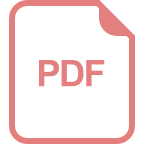
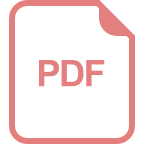