用pythpon写一个拼图游戏,其中可以实现自行插入图片
时间: 2024-03-06 12:47:23 浏览: 136
好的,我可以帮您提供一个带有图片功能的拼图游戏的 Python 代码。以下是代码:
```python
from tkinter import *
from tkinter import messagebox
from PIL import Image, ImageTk
import random
# 定义拼图的大小和形状
ROWS = 3
COLS = 3
BLANK_TILE = {"row": ROWS - 1, "col": COLS - 1}
TILE_WIDTH = 100
TILE_HEIGHT = 100
# 定义拼图块的编号和位置
tiles = []
for row in range(ROWS):
for col in range(COLS):
tile_num = row * COLS + col
tile = {"num": tile_num, "row": row, "col": col}
tiles.append(tile)
# 定义交换拼图块的函数
def swap_tiles(tile1, tile2):
temp = {"row": tile1["row"], "col": tile1["col"]}
tile1["row"] = tile2["row"]
tile1["col"] = tile2["col"]
tile2["row"] = temp["row"]
tile2["col"] = temp["col"]
# 定义打印拼图的函数
def print_puzzle():
for row in range(ROWS):
for col in range(COLS):
tile = get_tile(row, col)
if tile == BLANK_TILE:
print(" " * TILE_WIDTH, end="")
else:
print(str(tile["num"]).rjust(TILE_WIDTH), end="")
print()
# 定义获取拼图块的函数
def get_tile(row, col):
for tile in tiles:
if tile["row"] == row and tile["col"] == col:
return tile
return None
# 定义获取空拼图块的函数
def get_blank_tile():
return get_tile(BLANK_TILE["row"], BLANK_TILE["col"])
# 定义判断拼图是否完成的函数
def is_puzzle_solved():
for tile in tiles:
if tile["num"] != tile["row"] * COLS + tile["col"]:
return False
return True
# 定义交换拼图块的图像函数
def swap_tile_images(tile1, tile2):
temp_image = tile1["image"]
tile1["image"] = tile2["image"]
tile2["image"] = temp_image
tile1["button"].config(image=tile1["image"])
tile2["button"].config(image=tile2["image"])
# 定义打乱拼图的顺序函数
def shuffle_puzzle():
random.shuffle(tiles)
for row in range(ROWS):
for col in range(COLS):
tile = get_tile(row, col)
image = Image.open("puzzle.jpg")
box = (col * TILE_WIDTH, row * TILE_HEIGHT, (col + 1) * TILE_WIDTH, (row + 1) * TILE_HEIGHT)
tile_image = image.crop(box)
tile["image"] = ImageTk.PhotoImage(tile_image)
tile["button"].config(image=tile["image"])
# 定义开始游戏的函数
def start_game():
shuffle_puzzle()
moves = 0
while not is_puzzle_solved():
if messagebox.askyesno("Puzzle Game", "Are you sure you want to start a new game?"):
shuffle_puzzle()
moves = 0
else:
break
if is_puzzle_solved():
messagebox.showinfo("Puzzle Game", "Congratulations, you solved the puzzle in {} moves!".format(moves))
# 创建主窗口
root = Tk()
root.title("Puzzle Game")
# 加载拼图图片
image = Image.open("puzzle.jpg")
image_width, image_height = image.size
# 创建拼图块的按钮
for tile in tiles:
box = (tile["col"] * TILE_WIDTH, tile["row"] * TILE_HEIGHT, (tile["col"] + 1) * TILE_WIDTH, (tile["row"] + 1) * TILE_HEIGHT)
tile_image = image.crop(box)
tile["image"] = ImageTk.PhotoImage(tile_image)
tile["button"] = Button(root, image=tile["image"], command=lambda tile=tile: move_tile(tile))
tile["button"].grid(row=tile["row"], column=tile["col"])
# 创建开始游戏的按钮
start_button = Button(root, text="Start", command=start_game)
start_button.grid(row=ROWS, column=0, columnspan=COLS, pady=10)
# 定义移动拼图块的函数
def move_tile(tile):
blank_tile = get_blank_tile()
if tile["row"] == blank_tile["row"] and tile["col"] == blank_tile["col"] - 1:
# Move tile left
swap_tiles(tile, blank_tile)
swap_tile_images(tile, blank_tile)
elif tile["row"] == blank_tile["row"] and tile["col"] == blank_tile["col"] + 1:
# Move tile right
swap_tiles(tile, blank_tile)
swap_tile_images(tile, blank_tile)
elif tile["col"] == blank_tile["col"] and tile["row"] == blank_tile["row"] - 1:
# Move tile up
swap_tiles(tile, blank_tile)
swap_tile_images(tile, blank_tile)
elif tile["col"] == blank_tile["col"] and tile["row"] == blank_tile["row"] + 1:
# Move tile down
swap_tiles(tile, blank_tile)
swap_tile_images(tile, blank_tile)
else:
messagebox.showerror("Puzzle Game", "Invalid move!")
return
if is_puzzle_solved():
messagebox.showinfo("Puzzle Game", "Congratulations, you solved the puzzle!")
else:
moves = 0
for tile in tiles:
if tile["num"] != tile["row"] * COLS + tile["col"]:
moves += 1
messagebox.showinfo("Puzzle Game", "Moves: {}".format(moves))
# 运行主窗口
root.mainloop()
```
这个代码创建了一个 3x3 的拼图游戏,你可以根据需要修改游戏的大小。游戏开始时,代码会从 `puzzle.jpg` 文件中加载拼图图片,并将其分成若干个拼图块。玩家通过点击拼图块来移动它们,直到拼图完成。玩家可以点击 "Start" 按钮来开始游戏或重新开始游戏。在移动拼图块时,代码会交换相应的拼图块的位置和图像,并检查是否已完成拼图。如果拼图已完成,则代码会显示一个消息框来告诉玩家。如果拼图未完成,则代码会显示一个消息框来告诉玩家移动次数。
阅读全文
相关推荐





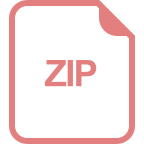
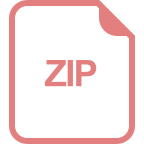
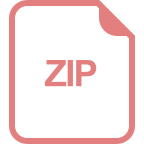
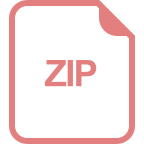
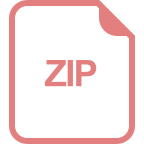
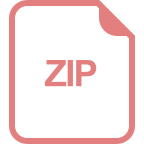
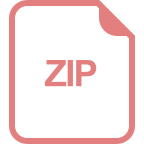
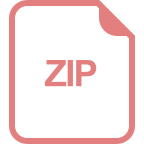