def rotate_points(x, y, x0, y0, phi):
时间: 2023-09-13 18:09:44 浏览: 63
这是一个函数,用于将点(x, y)绕点(x0, y0)旋转角度phi后的新坐标。其中x和y是点的原坐标,x0和y0是旋转中心的坐标,phi是旋转的角度。函数的实现可能类似于以下代码:
```
import math
def rotate_points(x, y, x0, y0, phi):
# 将角度转换为弧度
phi_rad = math.radians(phi)
# 计算旋转后的新坐标
x_new = (x - x0) * math.cos(phi_rad) - (y - y0) * math.sin(phi_rad) + x0
y_new = (x - x0) * math.sin(phi_rad) + (y - y0) * math.cos(phi_rad) + y0
# 返回旋转后的新坐标
return x_new, y_new
```
你可以通过调用这个函数来获得点(x, y)旋转后的新坐标。
相关问题
def rotate_points(x, y, x0, y0, phi):用c++实现
可以将上面的 Python 代码翻译成 C++ 代码,实现如下:
```c++
#include <cmath>
std::pair<double, double> rotate_points(double x, double y, double x0, double y0, double phi) {
// 将角度转换为弧度
double phi_rad = M_PI * phi / 180.0;
// 计算旋转后的新坐标
double x_new = (x - x0) * cos(phi_rad) - (y - y0) * sin(phi_rad) + x0;
double y_new = (x - x0) * sin(phi_rad) + (y - y0) * cos(phi_rad) + y0;
// 返回旋转后的新坐标
return std::make_pair(x_new, y_new);
}
```
在 C++ 代码中,使用 `#include <cmath>` 引入数学库,使用 `std::pair<double, double>` 表示返回值是一对 double 类型的值,使用 `std::make_pair(x_new, y_new)` 创建一对值。对于常量 pi,可以使用 `M_PI` 宏定义。
阅读全文
相关推荐
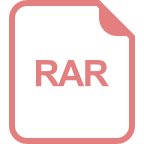
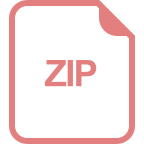
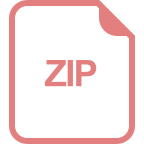
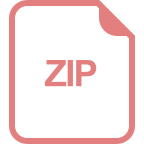
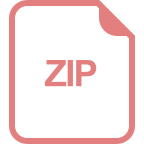
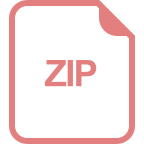
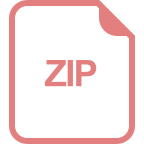
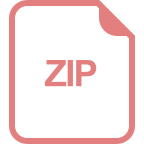
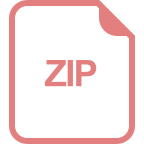
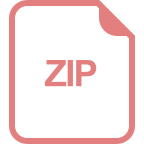
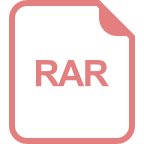
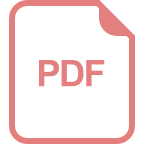
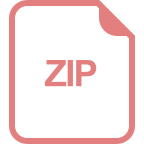
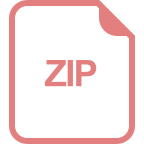
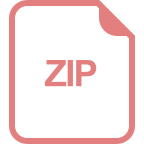
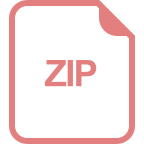
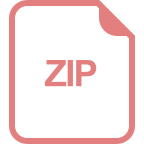