wxpython有哪些功能,请给出每一个模块的详细示例
时间: 2024-05-14 17:19:46 浏览: 10
wxPython 是 Python 编程语言的一种开发工具包(SDK),用于创建图形用户界面(GUI)应用程序。它基于wxWidgets C++ 类库,可以在多个平台上运行。下面是 wxPython 的常用功能和每个模块的示例:
1. wx.App : Gui 应用程序的主入口,用来启动程序并创建主窗口。
示例代码:
```
import wx
class MyFrame(wx.Frame):
def __init__(self, parent, title):
super(MyFrame, self).__init__(parent, title=title, size=(300, 200))
self.Show()
app = wx.App()
frame = MyFrame(None, 'Hello World')
app.MainLoop()
```
2. wx.Window : 所有用户界面的基本组件,用于创建应用程序中的各种视图组件。
示例代码:
```
import wx
class MyFrame(wx.Frame):
def __init__(self, parent, title):
super(MyFrame, self).__init__(parent, title=title, size=(300, 200))
self.panel = wx.Panel(self)
self.label = wx.StaticText(self.panel, label="Hello World!", pos=(50,50))
self.Show()
app = wx.App()
frame = MyFrame(None, 'Hello World')
app.MainLoop()
```
3. wx.Button : 创建一个常规按钮。
示例代码:
```
import wx
class MyFrame(wx.Frame):
def __init__(self, parent, title):
super(MyFrame, self).__init__(parent, title=title, size=(300, 200))
self.panel = wx.Panel(self)
self.button = wx.Button(self.panel, label="Click Me", pos=(50,50))
self.Show()
app = wx.App()
frame = MyFrame(None, 'Hello World')
app.MainLoop()
```
4. wx.TextCtrl : 创建一个文本框,用于输入或输出文本。
示例代码:
```
import wx
class MyFrame(wx.Frame):
def __init__(self, parent, title):
super(MyFrame, self).__init__(parent, title=title, size=(300, 200))
self.panel = wx.Panel(self)
self.tc = wx.TextCtrl(self.panel, pos=(50,50), size=(200, -1))
self.Show()
app = wx.App()
frame = MyFrame(None, 'Hello World')
app.MainLoop()
```
5. wx.ListBox : 创建一个列表框,用于显示并选择多个项目。
示例代码:
```
import wx
class MyFrame(wx.Frame):
def __init__(self, parent, title):
super(MyFrame, self).__init__(parent, title=title, size=(300, 200))
self.panel = wx.Panel(self)
self.lb = wx.ListBox(self.panel, choices=['item1', 'item2', 'item3'], pos=(50,50))
self.Show()
app = wx.App()
frame = MyFrame(None, 'Hello World')
app.MainLoop()
```
6. wx.MenuBar : 创建一个菜单栏,用于组织应用程序的主菜单。
示例代码:
```
import wx
class MyFrame(wx.Frame):
def __init__(self, parent, title):
super(MyFrame, self).__init__(parent, title=title, size=(300, 200))
self.panel = wx.Panel(self)
menubar = wx.MenuBar()
fileMenu = wx.Menu()
fileMenu.Append(wx.ID_OPEN, '&Open')
fileMenu.Append(wx.ID_SAVE, '&Save')
fileMenu.Append(wx.ID_EXIT, 'E&xit')
menubar.Append(fileMenu, '&File')
self.SetMenuBar(menubar)
self.Show()
app = wx.App()
frame = MyFrame(None, 'Hello World')
app.MainLoop()
```
7. wx.ToolBar : 创建一个工具条,用于快速访问应用程序中的常用功能。
示例代码:
```
import wx
class MyFrame(wx.Frame):
def __init__(self, parent, title):
super(MyFrame, self).__init__(parent, title=title, size=(300, 200))
self.panel = wx.Panel(self)
toolbar = self.CreateToolBar()
toolbar.AddLabelTool(wx.ID_NEW, 'New', wx.Bitmap('new.png'))
toolbar.AddLabelTool(wx.ID_OPEN, 'Open', wx.Bitmap('open.png'))
toolbar.Realize()
self.Show()
app = wx.App()
frame = MyFrame(None, 'Hello World')
app.MainLoop()
```
注:示例代码中使用的图片文件需要自己添加。
相关推荐
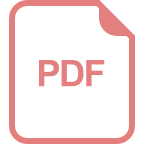
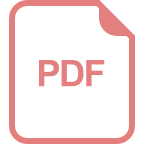
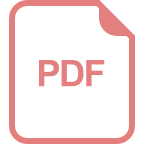














