vue+vuex+vuerouter+element+axios请求本地json文件实现增删改查
时间: 2023-07-29 15:09:38 浏览: 205
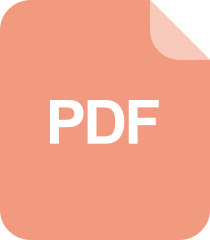
vue.js+Element实现表格里的增删改查
好的,下面是示例代码:
1. 在 src 目录下创建一个名为 data 的文件夹,在该文件夹内创建一个名为 data.json 的文件,用于存放数据。
2. 在 main.js 中引入相关库:
```javascript
import Vue from 'vue'
import App from './App.vue'
import Vuex from 'vuex'
import VueRouter from 'vue-router'
import ElementUI from 'element-ui'
import 'element-ui/lib/theme-chalk/index.css'
import axios from 'axios'
Vue.prototype.$axios = axios
Vue.config.productionTip = false
Vue.use(Vuex)
Vue.use(VueRouter)
Vue.use(ElementUI)
```
3. 在 store.js 中定义 Vuex 的状态管理:
```javascript
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
const store = new Vuex.Store({
state: {
data: [] // 存放数据的数组
},
mutations: {
setData(state, data) {
state.data = data
},
addData(state, newData) {
state.data.push(newData)
},
deleteData(state, id) {
const index = state.data.findIndex(item => item.id === id)
state.data.splice(index, 1)
},
updateData(state, newData) {
const index = state.data.findIndex(item => item.id === newData.id)
state.data.splice(index, 1, newData)
}
},
actions: {
async fetchData({ commit }) {
const response = await axios.get('/data/data.json')
commit('setData', response.data)
},
addData({ commit }, newData) {
commit('addData', newData)
},
deleteData({ commit }, id) {
commit('deleteData', id)
},
updateData({ commit }, newData) {
commit('updateData', newData)
}
}
})
export default store
```
4. 在 router.js 中定义路由:
```javascript
import Vue from 'vue'
import VueRouter from 'vue-router'
import Home from './views/Home.vue'
import Data from './views/Data.vue'
import AddData from './views/AddData.vue'
import EditData from './views/EditData.vue'
Vue.use(VueRouter)
const routes = [
{
path: '/',
name: 'home',
component: Home
},
{
path: '/data',
name: 'data',
component: Data
},
{
path: '/data/add',
name: 'addData',
component: AddData
},
{
path: '/data/edit/:id',
name: 'editData',
component: EditData
}
]
const router = new VueRouter({
mode: 'history',
base: process.env.BASE_URL,
routes
})
export default router
```
5. 在 App.vue 中使用 ElementUI 组件:
```html
<template>
<div id="app">
<el-header>
<el-menu :default-active="activeIndex" class="el-menu-demo" mode="horizontal" @select="handleSelect">
<el-menu-item index="/">首页</el-menu-item>
<el-menu-item index="/data">数据</el-menu-item>
</el-menu>
</el-header>
<el-main>
<router-view></router-view>
</el-main>
</div>
</template>
<script>
export default {
name: 'app',
computed: {
activeIndex() {
return this.$route.path
}
},
methods: {
handleSelect(index) {
this.$router.push(index)
}
}
}
</script>
```
6. 在 Data.vue 中显示数据列表,并实现删除操作:
```html
<template>
<div>
<el-table :data="data" style="width: 100%">
<el-table-column prop="id" label="ID"></el-table-column>
<el-table-column prop="name" label="姓名"></el-table-column>
<el-table-column prop="age" label="年龄"></el-table-column>
<el-table-column label="操作">
<template slot-scope="scope">
<el-button type="text" @click="handleEdit(scope.row)">编辑</el-button>
<el-button type="text" @click="handleDelete(scope.row.id)">删除</el-button>
</template>
</el-table-column>
</el-table>
<el-button type="primary" @click="handleAdd">添加</el-button>
</div>
</template>
<script>
export default {
name: 'data',
computed: {
data() {
return this.$store.state.data
}
},
methods: {
handleEdit(row) {
this.$router.push(`/data/edit/${row.id}`)
},
handleDelete(id) {
this.$confirm('确定删除该数据?', '提示', {
confirmButtonText: '确定',
cancelButtonText: '取消',
type: 'warning'
}).then(() => {
this.$store.dispatch('deleteData', id)
this.$message({
type: 'success',
message: '删除成功!'
})
}).catch(() => {})
},
handleAdd() {
this.$router.push('/data/add')
}
},
created() {
this.$store.dispatch('fetchData')
}
}
</script>
```
7. 在 AddData.vue 中实现添加操作:
```html
<template>
<div>
<el-form :model="formData" label-width="80px">
<el-form-item label="姓名">
<el-input v-model="formData.name"></el-input>
</el-form-item>
<el-form-item label="年龄">
<el-input v-model.number="formData.age"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="handleAdd">添加</el-button>
</el-form-item>
</el-form>
</div>
</template>
<script>
export default {
name: 'addData',
data() {
return {
formData: {
name: '',
age: ''
}
}
},
methods: {
handleAdd() {
const newData = { ...this.formData, id: Date.now() }
this.$store.dispatch('addData', newData)
this.$message({
type: 'success',
message: '添加成功!'
})
this.$router.push('/data')
}
}
}
</script>
```
8. 在 EditData.vue 中实现编辑操作:
```html
<template>
<div>
<el-form :model="formData" label-width="80px">
<el-form-item label="姓名">
<el-input v-model="formData.name"></el-input>
</el-form-item>
<el-form-item label="年龄">
<el-input v-model.number="formData.age"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="handleUpdate">更新</el-button>
</el-form-item>
</el-form>
</div>
</template>
<script>
export default {
name: 'editData',
data() {
return {
formData: {
id: '',
name: '',
age: ''
}
}
},
methods: {
handleUpdate() {
this.$store.dispatch('updateData', this.formData)
this.$message({
type: 'success',
message: '更新成功!'
})
this.$router.push('/data')
}
},
created() {
const id = this.$route.params.id
const data = this.$store.state.data.find(item => item.id === Number(id))
this.formData = data
}
}
</script>
```
以上就是一个简单的 Vue 前端实现增删改查的示例。需要注意的是,以上代码仅供参考,实际应用中需要根据具体需求进行修改。
阅读全文
相关推荐
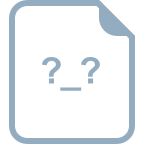
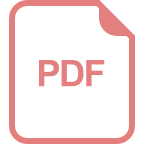

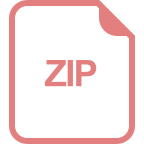
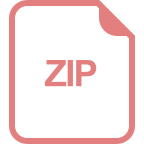
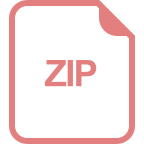
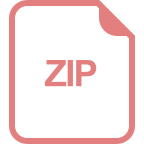
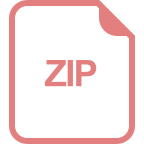
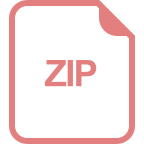
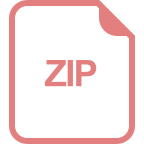
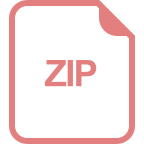
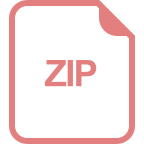
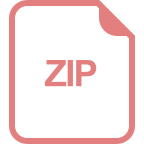
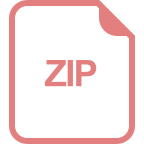
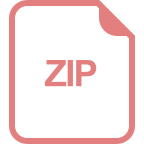
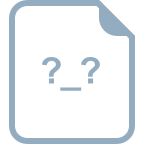
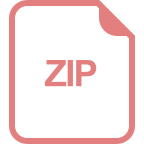
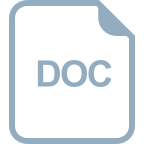