只用鏈表且不用algorithm實現支持負數的高精度運算(包括高精度乘高精度)
时间: 2023-08-21 09:19:33 浏览: 93
好的,我可以为您讲解一下高精度运算的实现方法。
首先,我们可以用链表来存储高精度数字,链表的每个节点存储一个数字位,链表的头结点存储最高位数字。对于负数,可以用一个布尔变量来表示正负。
接下来,我们可以实现加法运算。从低位到高位,依次将两个链表节点对应位置的数字相加,并将进位加到下一位相加。最后,如果最高位有进位,还需要在链表开头插入一个节点来存储进位。
高精度减法也可以通过加上相反数来实现。对于乘法,我们可以先实现单个数字和高精度数相乘的函数,再用类似于手算乘法的方法将两个高精度数相乘。
具体实现过程中,还需要注意一些细节,例如处理前导零,处理乘法结果中的进位等等。
下面是一个简单的 C++ 代码实现高精度加法和乘法的例子:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
struct ListNode {
int val;
ListNode *next;
ListNode(int x) : val(x), next(NULL) {}
};
class BigInt {
public:
BigInt() {
sign_ = false;
head_ = tail_ = nullptr;
}
BigInt(const char *str) {
sign_ = false;
head_ = tail_ = nullptr;
if (str[0] == '-') {
sign_ = true;
str++;
}
int len = strlen(str);
for (int i = len - 1; i >= 0; i--) {
ListNode *node = new ListNode(str[i] - '0');
if (head_ == nullptr) {
head_ = tail_ = node;
} else {
tail_->next = node;
tail_ = node;
}
}
}
void print() {
if (sign_) cout << '-';
ListNode *node = head_;
while (node != nullptr) {
cout << node->val;
node = node->next;
}
cout << endl;
}
BigInt operator+(const BigInt &rhs) {
BigInt ans;
ListNode *p = head_;
ListNode *q = rhs.head_;
int carry = 0;
while (p != nullptr || q != nullptr) {
int x = p ? p->val : 0;
int y = q ? q->val : 0;
int sum = x + y + carry;
carry = sum / 10;
ListNode *node = new ListNode(sum % 10);
if (ans.head_ == nullptr) {
ans.head_ = ans.tail_ = node;
} else {
ans.tail_->next = node;
ans.tail_ = node;
}
if (p) p = p->next;
if (q) q = q->next;
}
if (carry > 0) {
ListNode *node = new ListNode(carry);
ans.tail_->next = node;
ans.tail_ = node;
}
return ans;
}
BigInt operator*(const BigInt &rhs) {
BigInt ans;
ListNode *p = head_;
while (p != nullptr) {
int carry = 0;
ListNode *q = rhs.head_;
BigInt tmp;
while (q != nullptr) {
int x = p->val;
int y = q->val;
int prod = x * y + carry;
carry = prod / 10;
ListNode *node = new ListNode(prod % 10);
if (tmp.head_ == nullptr) {
tmp.head_ = tmp.tail_ = node;
} else {
tmp.tail_->next = node;
tmp.tail_ = node;
}
q = q->next;
}
if (carry > 0) {
ListNode *node = new ListNode(carry);
tmp.tail_->next = node;
tmp.tail_ = node;
}
tmp.tail_->next = nullptr;
for (int i = 0; i < ans.size(); i++) {
tmp.head_ = new ListNode(0);
}
ans = ans + tmp;
p = p->next;
}
ans.sign_ = sign_ ^ rhs.sign_;
return ans;
}
private:
bool sign_;
ListNode *head_;
ListNode *tail_;
};
int main() {
BigInt a("-123456789");
BigInt b("987654321");
BigInt c = a + b;
c.print();
BigInt d = a * b;
d.print();
return 0;
}
```
希望对您有所帮助!
阅读全文
相关推荐







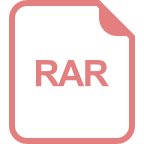


