实现论坛管理,在mysql中创建论坛表,包含留言时间、留言IP地址、留言内容并且在servlet程序中实现发表留言、留言显示、删除留言
时间: 2024-05-01 11:16:14 浏览: 15
在mysql中创建论坛表的SQL语句:
```
CREATE TABLE forum (
id INT AUTO_INCREMENT PRIMARY KEY,
post_time TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
ip_address VARCHAR(15) NOT NULL,
content TEXT NOT NULL
);
```
在servlet程序中实现发表留言、留言显示、删除留言的代码示例:
```java
import java.io.IOException;
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/ForumServlet")
public class ForumServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private static final String DB_URL = "jdbc:mysql://localhost:3306/forumdb?useSSL=false&serverTimezone=UTC";
private static final String DB_USER = "root";
private static final String DB_PASS = "password";
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String action = request.getParameter("action");
if (action == null) {
action = "list";
}
switch (action) {
case "new":
showNewForm(request, response);
break;
case "insert":
insertPost(request, response);
break;
case "delete":
deletePost(request, response);
break;
case "list":
default:
listPosts(request, response);
break;
}
}
private void listPosts(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
List<Post> postList = new ArrayList<>();
try (Connection conn = DriverManager.getConnection(DB_URL, DB_USER, DB_PASS);
PreparedStatement stmt = conn.prepareStatement("SELECT * FROM forum ORDER BY post_time DESC");
ResultSet rs = stmt.executeQuery()) {
while (rs.next()) {
Post post = new Post();
post.setId(rs.getInt("id"));
post.setPostTime(rs.getTimestamp("post_time"));
post.setIpAddress(rs.getString("ip_address"));
post.setContent(rs.getString("content"));
postList.add(post);
}
} catch (SQLException e) {
e.printStackTrace();
}
request.setAttribute("postList", postList);
request.getRequestDispatcher("forum.jsp").forward(request, response);
}
private void showNewForm(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.getRequestDispatcher("new_post.jsp").forward(request, response);
}
private void insertPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String ipAddress = request.getRemoteAddr();
String content = request.getParameter("content");
try (Connection conn = DriverManager.getConnection(DB_URL, DB_USER, DB_PASS);
PreparedStatement stmt = conn.prepareStatement("INSERT INTO forum (ip_address, content) VALUES (?, ?)")) {
stmt.setString(1, ipAddress);
stmt.setString(2, content);
stmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
response.sendRedirect("ForumServlet?action=list");
}
private void deletePost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int id = Integer.parseInt(request.getParameter("id"));
try (Connection conn = DriverManager.getConnection(DB_URL, DB_USER, DB_PASS);
PreparedStatement stmt = conn.prepareStatement("DELETE FROM forum WHERE id = ?")) {
stmt.setInt(1, id);
stmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
response.sendRedirect("ForumServlet?action=list");
}
}
```
在jsp页面中显示论坛留言的代码示例:
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Forum</title>
</head>
<body>
<h1>Forum</h1>
<a href="ForumServlet?action=new">New Post</a>
<br><br>
<table border="1">
<tr>
<th>ID</th>
<th>Date/Time</th>
<th>IP Address</th>
<th>Content</th>
<th>Action</th>
</tr>
<c:forEach items="${postList}" var="post">
<tr>
<td>${post.id}</td>
<td>${post.postTime}</td>
<td>${post.ipAddress}</td>
<td>${post.content}</td>
<td><a href="ForumServlet?action=delete&id=${post.id}">Delete</a></td>
</tr>
</c:forEach>
</table>
</body>
</html>
```
在jsp页面中发表新留言的代码示例:
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>New Post</title>
</head>
<body>
<h1>New Post</h1>
<form action="ForumServlet?action=insert" method="post">
<textarea name="content" rows="10" cols="50"></textarea>
<br>
<input type="submit" value="Post">
</form>
</body>
</html>
```
相关推荐
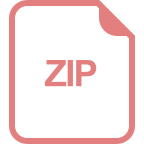
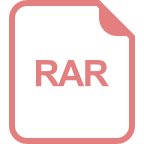
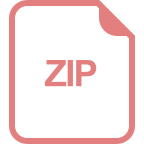








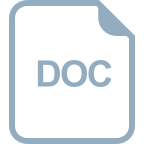
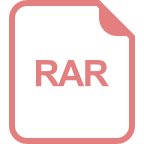
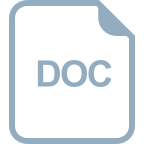
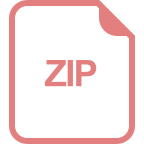
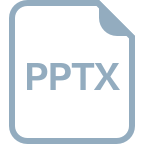
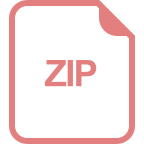
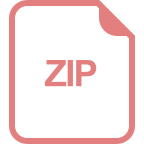