C# dotnetty 分包解决方案 客户端服务端的代码
时间: 2023-08-11 07:06:41 浏览: 62
以下是使用C# dotnetty实现分包解决方案的客户端和服务端示例代码:
### 服务端代码
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using DotNetty.Buffers;
using DotNetty.Codecs;
using DotNetty.Common.Utilities;
using DotNetty.Transport.Channels;
namespace Server
{
public class MessageDecoder : LengthFieldBasedFrameDecoder
{
public MessageDecoder() : base(ByteOrder.LittleEndian, 1024, 0, 4, 0, 4)
{
}
protected override Object Decode(IChannelHandlerContext ctx, IByteBuffer input)
{
var buffer = (IByteBuffer)base.Decode(ctx, input);
if (buffer == null)
{
return null;
}
var length = buffer.ReadInt();
var body = buffer.ReadBytes(length);
return new Request()
{
Length = length,
Body = body
};
}
}
public class MessageEncoder : MessageToByteEncoder<Response>
{
protected override void Encode(IChannelHandlerContext context, Response message, IByteBuffer output)
{
output.WriteInt(message.Length);
output.WriteBytes(message.Body);
}
}
public class ServerHandler : SimpleChannelInboundHandler<Request>
{
protected override void ChannelRead0(IChannelHandlerContext ctx, Request request)
{
Console.WriteLine($"Received message of length {request.Length}: {Encoding.UTF8.GetString(request.Body)}");
var response = new Response()
{
Length = request.Length,
Body = request.Body
};
ctx.WriteAsync(response);
}
public override void ExceptionCaught(IChannelHandlerContext context, Exception exception)
{
Console.WriteLine($"Exception: {exception}");
context.CloseAsync();
}
}
public class Request
{
public int Length { get; set; }
public byte[] Body { get; set; }
}
public class Response
{
public int Length { get; set; }
public byte[] Body { get; set; }
}
}
```
### 客户端代码
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using DotNetty.Buffers;
using DotNetty.Codecs;
using DotNetty.Common.Utilities;
using DotNetty.Transport.Channels;
namespace Client
{
public class MessageDecoder : LengthFieldBasedFrameDecoder
{
public MessageDecoder() : base(ByteOrder.LittleEndian, 1024, 0, 4, 0, 4)
{
}
protected override Object Decode(IChannelHandlerContext ctx, IByteBuffer input)
{
var buffer = (IByteBuffer)base.Decode(ctx, input);
if (buffer == null)
{
return null;
}
var length = buffer.ReadInt();
var body = buffer.ReadBytes(length);
return new Response()
{
Length = length,
Body = body
};
}
}
public class MessageEncoder : MessageToByteEncoder<Request>
{
protected override void Encode(IChannelHandlerContext context, Request message, IByteBuffer output)
{
output.WriteInt(message.Length);
output.WriteBytes(message.Body);
}
}
public class ClientHandler : SimpleChannelInboundHandler<Response>
{
private readonly TaskCompletionSource<Response> _responsePromise;
public ClientHandler(TaskCompletionSource<Response> responsePromise)
{
_responsePromise = responsePromise;
}
protected override void ChannelRead0(IChannelHandlerContext ctx, Response response)
{
Console.WriteLine($"Received message of length {response.Length}: {Encoding.UTF8.GetString(response.Body)}");
_responsePromise.TrySetResult(response);
}
public override void ExceptionCaught(IChannelHandlerContext context, Exception exception)
{
Console.WriteLine($"Exception: {exception}");
context.CloseAsync();
}
}
public class Request
{
public int Length { get; set; }
public byte[] Body { get; set; }
}
public class Response
{
public int Length { get; set; }
public byte[] Body { get; set; }
}
}
```
相关推荐
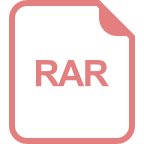
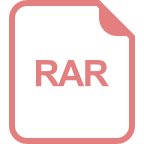
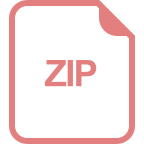














