def max_occurrence(s): # 构建哈希表记录每个字母出现的次数 count = {} for c in s: count[c] = count.get(c, 0) + 1 # 找到出现次数最多的字母及其出现次数 max_count = 0 max_chars = [] for c in count: if count[c] > max_count: max_count = count[c] max_chars = [c] elif count[c] == max_count: max_chars.append(c) # 对出现次数一样的字母按字符从小到大排序 max_chars.sort() # 返回结果 return max_chars[0], max_count将这段代码变简单
时间: 2023-06-20 21:05:05 浏览: 40
可以使用Python内置的collections模块中的Counter类来替代手写的哈希表统计每个字母出现的次数,然后使用max函数来找到出现次数最多的字母及其出现次数,最后使用sorted函数对出现次数一样的字母按字符从小到大排序。代码如下:
from collections import Counter
def max_occurrence(s):
count = Counter(s)
max_count = max(count.values())
max_chars = sorted([c for c in count if count[c] == max_count])
return max_chars[0], max_count
相关问题
def max_occurrence(s): # 构建哈希表记录每个字母出现的次数 count = {} for c in s: count[c] = count.get(c, 0) + 1 # 找到出现次数最多的字母及其出现次数 max_count = 0 max_chars = [] for c in count: if count[c] > max_count: max_count = count[c] max_chars = [c] elif count[c] == max_count: max_chars.append(c) # 对出现次数一样的字母按字符从小到大排序 max_chars.sort() # 返回结果 return max_chars[0], max_count将这段代码不使用函数
以下是不使用函数的代码实现:
```python
s = input("请输入一个字符串:")
# 构建哈希表记录每个字母出现的次数
count = {}
for c in s:
count[c] = count.get(c, 0) + 1
# 找到出现次数最多的字母及其出现次数
max_count = 0
max_chars = []
for c in count:
if count[c] > max_count:
max_count = count[c]
max_chars = [c]
elif count[c] == max_count:
max_chars.append(c)
# 对出现次数一样的字母按字符从小到大排序
max_chars.sort()
# 返回结果
print("出现次数最多的字母是:{},出现次数为:{}".format(max_chars[0], max_count))
```
输入字符串,输出字符串中出现次数最多的字母及其出现次数,如果有多个字母出现次数一样,按字符从小到大顺序输出字母及其出现次数
可以使用哈希表来记录每个字母出现的次数,然后遍历哈希表找到出现次数最多的字母及其出现次数。如果有多个字母出现次数一样,可以将哈希表按键值(即字母)从小到大排序后输出。
以下是 Python 代码实现:
```python
def max_occurrence(s):
# 构建哈希表记录每个字母出现的次数
count = {}
for c in s:
count[c] = count.get(c, 0) + 1
# 找到出现次数最多的字母及其出现次数
max_count = 0
max_chars = []
for c in count:
if count[c] > max_count:
max_count = count[c]
max_chars = [c]
elif count[c] == max_count:
max_chars.append(c)
# 对出现次数一样的字母按字符从小到大排序
max_chars.sort()
# 返回结果
return max_chars[0], max_count
```
测试:
```python
s = 'hello world'
print(max_occurrence(s)) # ('l', 3)
s = 'abcbcdcde'
print(max_occurrence(s)) # ('c', 3)
```
相关推荐
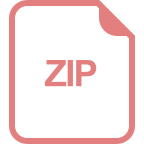
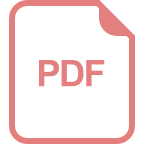













