c#sql server queryout 如何导出到文件夹
时间: 2024-04-09 22:33:25 浏览: 208
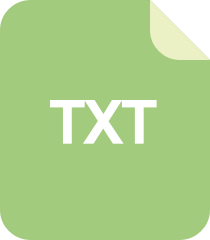
sqlserver bcp导出到excel

在 C# 中,你可以使用 `SqlBulkCopy` 类将 SQL Server 中的查询结果导出到文件夹。下面是一个示例代码:
```csharp
using System.Data.SqlClient;
// 数据库连接字符串
string connectionString = "Data Source=YourServer;Initial Catalog=YourDatabase;User ID=YourUsername;Password=YourPassword";
// 查询语句
string query = "SELECT * FROM YourTable";
// 导出文件路径和文件名
string filePath = @"C:\YourFolder\YourFile.csv";
// 创建 SQL 连接对象
using (SqlConnection connection = new SqlConnection(connectionString))
{
// 打开数据库连接
connection.Open();
// 创建 SQL 命令对象
using (SqlCommand command = new SqlCommand(query, connection))
{
// 使用 SqlDataReader 读取查询结果
using (SqlDataReader reader = command.ExecuteReader())
{
// 创建 CSV 文件写入流
using (System.IO.StreamWriter file = new System.IO.StreamWriter(filePath))
{
// 写入列名
for (int i = 0; i < reader.FieldCount; i++)
{
file.Write(reader.GetName(i));
if (i < reader.FieldCount - 1)
file.Write(",");
}
file.WriteLine();
// 写入数据行
while (reader.Read())
{
for (int i = 0; i < reader.FieldCount; i++)
{
file.Write(reader[i]);
if (i < reader.FieldCount - 1)
file.Write(",");
}
file.WriteLine();
}
}
}
}
}
```
上述代码使用 `SqlConnection` 建立与 SQL Server 的连接,使用 `SqlCommand` 执行查询语句,并使用 `SqlDataReader` 读取查询结果。然后,将结果逐行写入到指定的文件路径中。请确保指定的文件夹路径存在,并且对该路径有写入权限。
阅读全文
相关推荐
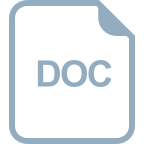
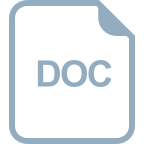

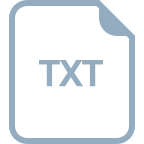
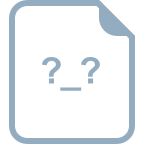
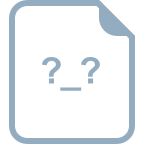
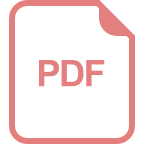
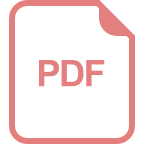
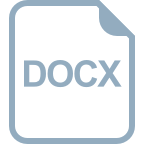
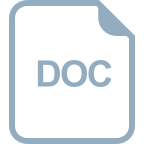
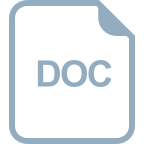
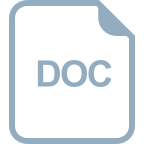




