processing制作粒子效果
时间: 2023-11-25 14:05:22 浏览: 131
要制作粒子效果,使用Processing可以使用两种方式:使用现有的粒子库或自己编写代码。
1. 使用现有的粒子库:Processing有许多粒子库可供使用,如Toxiclibs、Traer等。这些库提供了许多现成的函数和类,可以轻松地创建和控制粒子效果。
例如,使用Toxiclibs库可以轻松地创建一个简单的粒子效果:
```
import toxi.geom.*;
import toxi.physics.*;
import toxi.physics.behaviors.*;
VerletPhysics physics;
VerletParticle[] particles;
void setup() {
size(400, 400);
physics = new VerletPhysics();
particles = new VerletParticle[100];
for (int i = 0; i < particles.length; i++) {
particles[i] = new VerletParticle(new Vec2D(random(width), random(height)));
physics.addParticle(particles[i]);
physics.addBehavior(new AttractionBehavior(particles[i], 20, -1));
}
}
void draw() {
background(255);
physics.update();
stroke(0);
for (int i = 0; i < particles.length; i++) {
point(particles[i].x, particles[i].y);
}
}
```
2. 自己编写代码:如果你想更深入地了解粒子效果的原理和实现方法,可以自己编写代码。通常需要使用向量、物理引擎等概念和技术。
例如,可以使用向量来表示粒子的位置和速度,使用物理引擎来模拟粒子的运动和碰撞:
```
Particle[] particles;
void setup() {
size(400, 400);
particles = new Particle[100];
for (int i = 0; i < particles.length; i++) {
particles[i] = new Particle(new PVector(random(width), random(height)), new PVector(random(-1, 1), random(-1, 1)));
}
}
void draw() {
background(255);
for (int i = 0; i < particles.length; i++) {
particles[i].update();
particles[i].display();
}
}
class Particle {
PVector pos, vel, acc;
Particle(PVector pos, PVector vel) {
this.pos = pos;
this.vel = vel;
this.acc = new PVector(0, 0.1);
}
void update() {
vel.add(acc);
pos.add(vel);
if (pos.x < 0 || pos.x > width) vel.x *= -1;
if (pos.y < 0 || pos.y > height) vel.y *= -1;
}
void display() {
stroke(0);
ellipse(pos.x, pos.y, 10, 10);
}
}
```
以上是两种制作粒子效果的方法,你可以根据自己的需求和技能选择适合自己的方式。
阅读全文
相关推荐
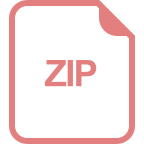

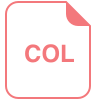

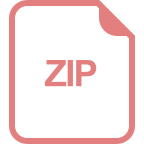
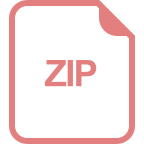
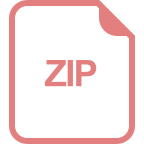
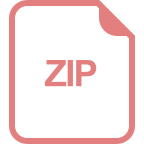
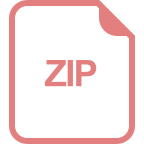
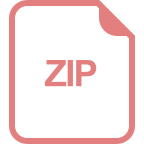
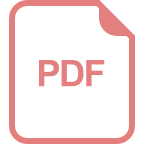
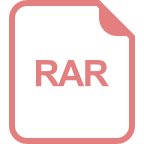
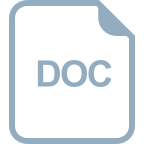
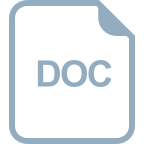
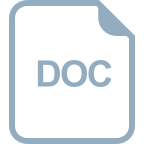
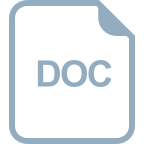
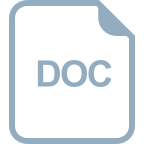