OpenCV 库中的 VideoCapture 类来读取视频文件进行光流法视频追踪c#
时间: 2023-12-25 08:16:27 浏览: 232
在 C# 中使用 OpenCV 库进行光流法视频追踪的方法与 C++ 中类似,只是语法上有所不同。需要使用 NuGet 包管理器安装 EmguCV 库,它是 C# 的 OpenCV 库封装。
代码示例:
```
using System;
using Emgu.CV;
using Emgu.CV.Structure;
using Emgu.CV.Util;
using Emgu.CV.Features2D;
namespace OpticalFlowDemo
{
class Program
{
static void Main(string[] args)
{
// 读取视频文件
VideoCapture capture = new VideoCapture("test.avi");
if (!capture.IsOpened)
{
Console.WriteLine("Error opening video file");
return;
}
// 获取第一帧图像
Mat prev_frame = new Mat();
Mat next_frame = new Mat();
capture.Read(prev_frame);
// 转换为灰度图像
CvInvoke.CvtColor(prev_frame, prev_frame, Emgu.CV.CvEnum.ColorConversion.Bgr2Gray);
// 提取关键点
VectorOfPointF prev_points = new VectorOfPointF();
VectorOfPointF next_points = new VectorOfPointF();
GFTTDetector detector = new GFTTDetector(100, 0.3, 7, 7, false, 0.04);
detector.Detect(prev_frame, prev_points);
// 进行追踪
Mat status = new Mat();
Mat err = new Mat();
while (true)
{
// 读取下一帧图像
capture.Read(next_frame);
if (next_frame.IsEmpty)
break;
// 转换为灰度图像
CvInvoke.CvtColor(next_frame, next_frame, Emgu.CV.CvEnum.ColorConversion.Bgr2Gray);
// 进行光流法追踪
OpticalFlow.PyrLK(prev_frame, next_frame, prev_points, next_points, status, err);
// 绘制轨迹
PointF[] prev_points_array = prev_points.ToArray();
PointF[] next_points_array = next_points.ToArray();
for (int i = 0; i < prev_points_array.Length; i++)
{
if (status.GetData(i)[0] == 1)
{
CvInvoke.Line(next_frame, Point.Round(prev_points_array[i]), Point.Round(next_points_array[i]), new MCvScalar(0, 255, 0), 2);
CvInvoke.Circle(next_frame, Point.Round(next_points_array[i]), 5, new MCvScalar(0, 0, 255), -1);
}
}
// 显示图像
CvInvoke.Imshow("Optical Flow Tracking", next_frame);
// 更新变量
prev_points = next_points;
prev_frame = next_frame.Clone();
// 等待按键
if (CvInvoke.WaitKey(30) == 27)
break;
}
// 释放资源
capture.Dispose();
CvInvoke.DestroyAllWindows();
}
}
}
```
阅读全文
相关推荐




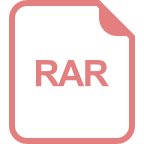
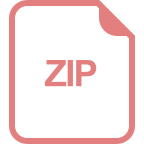
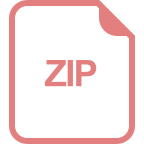
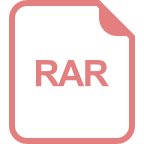
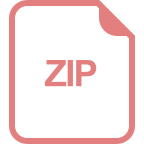
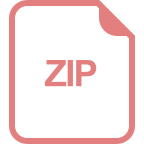
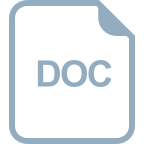
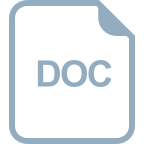
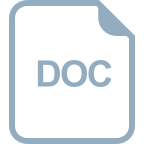
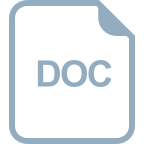
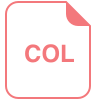
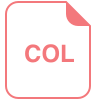