用C++帮我写一个串的赋值函数
时间: 2023-05-27 18:02:41 浏览: 82
以下是一个示例串的赋值函数,基于C语言:
```
#include <stdio.h>
#include <string.h>
void assign(char* destination, char* source) {
int i;
for (i = 0; i < strlen(source); i++) {
destination[i] = source[i];
}
destination[i] = '\0';
}
int main() {
char string1[20];
char string2[] = "Hello, World!";
assign(string1, string2);
printf("string1: %s\n", string1);
printf("string2: %s\n", string2);
return 0;
}
```
在本例中,`assign`函数接收一个指向目标串的指针和一个指向源串的指针,并通过遍历源串将其复制到目标串中。最后,函数通过在目标串的结尾添加一个null终止符来确保新的串正确地结束。
在`main`函数中,我们声明了两个串`string1`和`string2`,并将`string2`传递给`assign`函数,将其赋值到`string1`。
相关问题
C++ string 赋值函数 移动赋值函数 移动构造函数
C++中的字符串赋值函数、移动赋值函数和移动构造函数分别是:
1. 字符串赋值函数(Assignment Operator):在C++中,可以使用赋值操作符(=)来将一个字符串赋值给另一个字符串。例如:
```cpp
std::string str1 = "Hello";
std::string str2;
str2 = str1; // 使用赋值操作符将str1的值赋给str2
```
这里,使用赋值操作符将str1的值赋给str2。
2. 移动赋值函数(Move Assignment Operator):移动赋值函数用于在性能上优化对象的赋值过程,通过移动资源而不是进行深拷贝。它使用`&&`来接收右值引用参数。例如:
```cpp
class MyString {
public:
// 移动赋值函数
MyString& operator=(MyString&& other) noexcept {
if (this != &other) {
// 释放当前对象的资源
delete[] m_data;
// 移动other的资源到当前对象
m_data = other.m_data;
m_size = other.m_size;
// 清空other对象
other.m_data = nullptr;
other.m_size = 0;
}
return *this;
}
private:
char* m_data;
size_t m_size;
};
```
这里,移动赋值函数通过移动`other`对象的资源到当前对象,并清空`other`对象。
3. 移动构造函数(Move Constructor):移动构造函数用于在性能上优化对象的构造过程,通过移动资源而不是进行深拷贝。它使用`&&`来接收右值引用参数。例如:
```cpp
class MyString {
public:
// 移动构造函数
MyString(MyString&& other) noexcept {
m_data = other.m_data;
m_size = other.m_size;
other.m_data = nullptr;
other.m_size = 0;
}
private:
char* m_data;
size_t m_size;
};
```
这里,移动构造函数通过移动`other`对象的资源到当前对象,并清空`other`对象。
需要注意的是,移动赋值函数和移动构造函数一般需要保证不抛出异常,因此通常会使用`noexcept`关键字声明它们。另外,移动赋值函数和移动构造函数通常与移动语义相关的类一起使用,如智能指针、容器等。
c++字符串赋值给字符串
要将一个 C++ 字符串赋值给另一个字符串,可以使用赋值运算符“=”。例如,假设有两个字符串变量 str1 和 str2,要将 str2 的值赋给 str1,可以这样写:
```
str1 = str2;
```
这会将 str2 的内容复制到 str1 中。注意,这只适用于 C++ 字符串类型,而不是 C 语言中的字符数组。如果要将字符数组赋值给 C++ 字符串,可以使用字符串构造函数,例如:
```
char arr[] = "Hello";
std::string str(arr);
```
这会创建一个新的字符串 str,其值为 "Hello"。
阅读全文
相关推荐
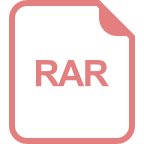
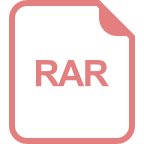
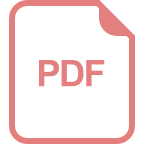
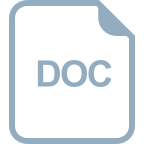
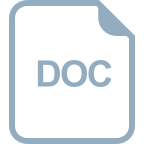
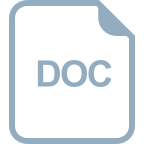
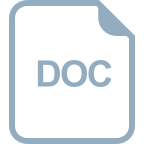
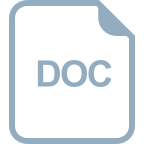
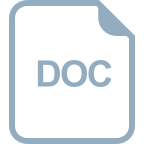
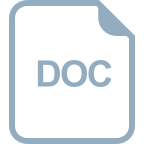
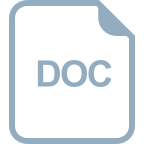
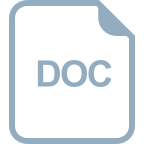
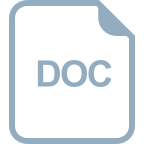

