AES128C代码
时间: 2023-07-02 11:10:57 浏览: 92
以下是使用AES128C加密的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/aes.h>
#include <openssl/rand.h>
#define AES_KEY_LENGTH 128
int main()
{
unsigned char key[AES_KEY_LENGTH/8];
unsigned char iv[AES_BLOCK_SIZE];
// 生成随机密钥和IV
RAND_bytes(key, sizeof(key));
RAND_bytes(iv, sizeof(iv));
// 声明AES加密上下文
AES_KEY aes_key;
// 初始化加密上下文
AES_set_encrypt_key(key, AES_KEY_LENGTH, &aes_key);
// 明文
unsigned char plaintext[] = "Hello World!";
// 计算明文长度并分配加密缓冲区
int plaintext_len = strlen((char *)plaintext);
int ciphertext_len = ((plaintext_len + AES_BLOCK_SIZE - 1) / AES_BLOCK_SIZE) * AES_BLOCK_SIZE;
unsigned char *ciphertext = (unsigned char *)malloc(ciphertext_len);
// AES加密
AES_cbc_encrypt(plaintext, ciphertext, plaintext_len, &aes_key, iv, AES_ENCRYPT);
// 输出密文
printf("Ciphertext: ");
for(int i=0; i<ciphertext_len; i++)
printf("%02x", ciphertext[i]);
printf("\n");
// 释放加密缓冲区
free(ciphertext);
return 0;
}
```
注意,此示例使用OpenSSL库进行AES加密。在使用前,确保已正确安装和配置OpenSSL库。
阅读全文
相关推荐

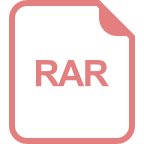













