opencv qt实现多张图片全景拼接源码
时间: 2023-12-07 10:02:18 浏览: 78
以下是使用OpenCV和Qt实现多张图片全景拼接的示例代码:
```cpp
#include <opencv2/opencv.hpp>
#include <QImage>
#include <QPixmap>
#include <QFileDialog>
#include <QMessageBox>
using namespace cv;
void stitchImages(const std::vector<Mat>& images, Mat& result)
{
// Create a Stitcher object
cv::Ptr<Stitcher> stitcher = Stitcher::create(Stitcher::PANORAMA);
// Stitch the images
Stitcher::Status status = stitcher->stitch(images, result);
if (status != Stitcher::OK)
{
QMessageBox::warning(nullptr, "Error", "Failed to stitch images!");
}
}
QImage matToQImage(const cv::Mat& mat)
{
if (mat.type() == CV_8UC1)
{
// Grayscale image
return QImage(mat.data, mat.cols, mat.rows, static_cast<int>(mat.step), QImage::Format_Grayscale8);
}
else if (mat.type() == CV_8UC3)
{
// Color image
cv::Mat rgbMat;
cv::cvtColor(mat, rgbMat, cv::COLOR_BGR2RGB);
return QImage(rgbMat.data, rgbMat.cols, rgbMat.rows, static_cast<int>(rgbMat.step), QImage::Format_RGB888);
}
else
{
QMessageBox::warning(nullptr, "Error", "Unknown image type!");
return QImage();
}
}
int main(int argc, char** argv)
{
// Read the input images
QStringList fileNames = QFileDialog::getOpenFileNames(nullptr, "Select Images", ".", "Images (*.png *.jpg *.jpeg)");
if (fileNames.isEmpty())
{
return 0;
}
std::vector<cv::Mat> images;
for (const auto& fileName : fileNames)
{
cv::Mat image = cv::imread(fileName.toStdString());
if (image.empty())
{
QMessageBox::warning(nullptr, "Error", "Failed to read image!");
return 0;
}
images.push_back(image);
}
// Stitch the images
cv::Mat result;
stitchImages(images, result);
// Convert the result to a QImage and display it
QImage resultImage = matToQImage(result);
if (resultImage.isNull())
{
QMessageBox::warning(nullptr, "Error", "Failed to convert image!");
return 0;
}
QPixmap pixmap = QPixmap::fromImage(resultImage);
QLabel label;
label.setPixmap(pixmap);
label.show();
return QApplication(argc, argv).exec();
}
```
这个示例代码首先使用Qt的文件对话框选择输入图像,然后使用OpenCV的 `Stitcher` 类将所有图像拼接成一个全景图像。最后,将结果转换为 `QImage` 并使用 `QLabel` 显示。
相关推荐
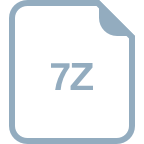
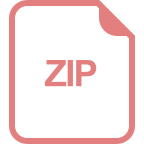














