ffmpeg_g -re -i /home/tsdl/Downloads/FPV_2021-0-1_12-37-23.h264 -vcodec libx264 -profile:v baseline -r 25 -g 50 -f rtc "http://192.168.114.34:1985/rtc/v1/whip/?app=live&stream=livestream"转化成C语言代码
时间: 2023-12-16 22:04:53 浏览: 36
将FFmpeg命令转化为C语言代码需要使用FFmpeg的C语言API,下面是一个简单的示例:
```c
#include <stdio.h>
#include <libavformat/avformat.h>
#include <libavutil/opt.h>
int main(int argc, char *argv[]) {
int ret;
AVFormatContext *in_fmt_ctx = NULL;
AVCodecContext *in_codec_ctx = NULL;
AVOutputFormat *out_fmt = NULL;
AVFormatContext *out_fmt_ctx = NULL;
AVStream *in_stream = NULL;
AVStream *out_stream = NULL;
AVPacket pkt;
// 打开输入文件
const char *input_file = "/home/tsdl/Downloads/FPV_2021-0-1_12-37-23.h264";
if ((ret = avformat_open_input(&in_fmt_ctx, input_file, NULL, NULL)) < 0) {
fprintf(stderr, "Could not open input file '%s'\n", input_file);
goto end;
}
// 获取输入流信息
if ((ret = avformat_find_stream_info(in_fmt_ctx, NULL)) < 0) {
fprintf(stderr, "Failed to retrieve input stream information\n");
goto end;
}
// 获取输入视频流
int video_stream_index = -1;
for (int i = 0; i < in_fmt_ctx->nb_streams; i++) {
if (in_fmt_ctx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
video_stream_index = i;
break;
}
}
if (video_stream_index == -1) {
fprintf(stderr, "Input file does not contain a video stream\n");
goto end;
}
in_stream = in_fmt_ctx->streams[video_stream_index];
in_codec_ctx = avcodec_alloc_context3(NULL);
if (!in_codec_ctx) {
fprintf(stderr, "Failed to allocate codec context\n");
goto end;
}
if ((ret = avcodec_parameters_to_context(in_codec_ctx, in_stream->codecpar)) < 0) {
fprintf(stderr, "Failed to copy codec parameters to codec context\n");
goto end;
}
// 打开输出URL
const char *output_url = "http://192.168.114.34:1985/rtc/v1/whip/?app=live&stream=livestream";
if ((ret = avformat_alloc_output_context2(&out_fmt_ctx, NULL, "rtc", output_url)) < 0) {
fprintf(stderr, "Could not allocate output format context\n");
goto end;
}
out_fmt = out_fmt_ctx->oformat;
// 添加输出流
out_stream = avformat_new_stream(out_fmt_ctx, NULL);
if (!out_stream) {
fprintf(stderr, "Failed to create new output stream\n");
goto end;
}
AVCodecParameters *codecpar = out_stream->codecpar;
codecpar->codec_type = AVMEDIA_TYPE_VIDEO;
codecpar->codec_id = AV_CODEC_ID_H264;
codecpar->width = in_codec_ctx->width;
codecpar->height = in_codec_ctx->height;
codecpar->format = in_codec_ctx->pix_fmt;
avcodec_parameters_to_context(out_stream->codec, codecpar);
// 打开输出URL
if (!(out_fmt->flags & AVFMT_NOFILE)) {
if ((ret = avio_open(&out_fmt_ctx->pb, output_url, AVIO_FLAG_WRITE)) < 0) {
fprintf(stderr, "Could not open output URL '%s'\n", output_url);
goto end;
}
}
// 写输出文件头
if ((ret = avformat_write_header(out_fmt_ctx, NULL)) < 0) {
fprintf(stderr, "Error occurred when writing output file header\n");
goto end;
}
// 转码并输出
while (1) {
ret = av_read_frame(in_fmt_ctx, &pkt);
if (ret < 0) {
break;
}
if (pkt.stream_index == video_stream_index) {
av_interleaved_write_frame(out_fmt_ctx, &pkt);
}
av_packet_unref(&pkt);
}
// 写输出文件尾
av_write_trailer(out_fmt_ctx);
end:
if (in_fmt_ctx) {
avformat_close_input(&in_fmt_ctx);
}
if (in_codec_ctx) {
avcodec_free_context(&in_codec_ctx);
}
if (out_fmt_ctx) {
if (out_fmt_ctx->pb) {
avio_closep(&out_fmt_ctx->pb);
}
avformat_free_context(out_fmt_ctx);
}
return ret;
}
```
上面的代码使用了FFmpeg的C语言API来打开输入文件、获取输入流信息、获取输入视频流、打开输出URL、添加输出流、转码并输出、写输出文件头和输出文件尾等操作。请注意,此处的代码仅供参考,具体实现可能需要根据实际情况进行调整。
相关推荐
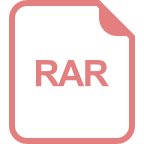
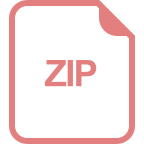
















