编写一个函数isGoodpwd(s),检查传入的字符串s是否是一个好密码,返回True或False。好密码的标准:密码长度>=8, 只能由字母和数字构成,且必须同时含有大小写字母和数字,不能含有空格和标点符号等特殊字符。
时间: 2024-05-09 13:17:58 浏览: 7
好的,这是一个程序问题,我可以回答。以下是一个Python的实现:
```python
def isGoodpwd(s):
if len(s) < 8:
return False
if not any(c.isupper() for c in s):
return False
if not any(c.islower() for c in s):
return False
if not any(c.isdigit() for c in s):
return False
if any(c.isspace() or not c.isalnum() for c in s):
return False
return True
```
这个函数首先检查密码的长度是否大于等于8,然后分别检查密码是否包含大小写字母和数字,最后检查密码是否只包含字母和数字(不包含空格和特殊字符)。
相关问题
编写一个函数,判断传入的字符串是否为“回文联”,如果是,返回“true”,否则,返回“false”
以下是 Python 代码实现:
```python
def is_palindrome(text):
# 去掉所有空格和标点符号,并将所有字母转换为小写
text = ''.join(ch.lower() for ch in text if ch.isalnum())
# 判断字符串是否等于它的反转
return text == text[::-1]
# 测试
print(is_palindrome('上海自来水来自海上')) # True
print(is_palindrome('A man, a plan, a canal, Panama!')) # True
print(is_palindrome('Hello, world!')) # False
```
该函数会首先将传入的字符串中所有的非数字和字母的字符都去掉,并将剩下的字符转换为小写。然后,它会判断该字符串是否等于它的反转。如果是,就说明它是回文联,返回 True,否则返回 False。
编写一函数测试一个字符串是否是回文串
好的,我可以为您编写一个测试字符串是否是回文串的函数。以下是Python代码示例:
```python
def is_palindrome(string):
"""
判断给定字符串是否是回文串
"""
i, j = 0, len(string) - 1
while i < j:
if string[i] != string[j]:
return False
i += 1
j -= 1
return True
```
您可以将要测试的字符串作为函数的参数传入,函数将返回一个布尔值,表明这个字符串是否是一个回文串。
相关推荐
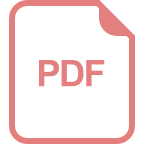
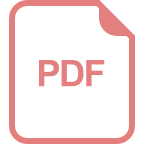
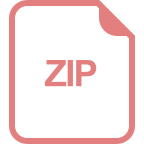












