【问题描述】 从文件in.txt读取信息,文件里的单词中间由空格隔开 统计每个单词出现的次数,从大到小排序后输出到文件out.txt 【输入形式】 文件里存储了多个单词,有重复,空格隔开 【输出形式】 从大到小输出单词及其出现的次数,次数一样的单词按字符串比较从小到大排序 【输入样例】in.txt文件里面的数据如下所示: hello world python hello word file number file file 【输出样例】out.txt文件里面的数据如下所示: file 3 hello 2 number 1 python 1 word 1 world 1
时间: 2024-03-21 08:43:51 浏览: 9
这个问题可以使用Python的文件读写操作和字典处理来实现。代码如下:
```python
# 读取文件内容
with open("in.txt", "r") as f:
content = f.read()
# 将文本文件中的单词转换为列表
words = content.split()
# 使用字典统计每个单词出现的次数
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
# 按照出现次数从大到小排序,次数一样的单词按照字符串从小到大排序
sorted_word_count = sorted(word_count.items(), key=lambda x: (-x[1], x[0]))
# 将结果写入文件
with open("out.txt", "w") as f:
for word, count in sorted_word_count:
f.write(word + " " + str(count) + "\n")
```
这个程序会读取当前目录下的 in.txt 文件中的内容,并将其中的单词转换为列表。然后使用字典统计每个单词出现的次数。最后使用sorted函数按照出现次数从大到小排序,次数一样的单词按照字符串从小到大排序。
排序结果是一个元组列表,每个元组包含单词和出现次数。最后将结果写入到 out.txt 文件中。
注意,如果文件中的单词包含标点符号或换行符等非单词字符,需要在转换为列表之前进行判断和过滤。
相关推荐
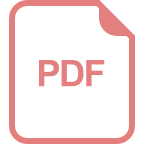
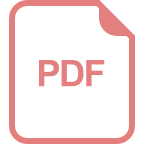
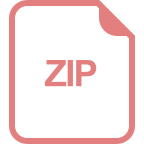












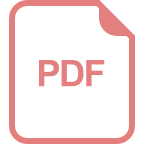
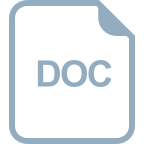