qt删除二进制文件后面一定长度,用c++实现
时间: 2023-12-03 15:42:26 浏览: 35
可以使用C++中的文件流(fstream)来实现。
首先,打开二进制文件并定位到需要删除的位置,然后截取文件内容。最后,将截取后的内容写回到原文件中。
示例代码如下:
```cpp
#include <fstream>
#include <iostream>
using namespace std;
int main() {
const string filename = "binary_file.bin"; // 二进制文件名
const int len_to_delete = 10; // 需要删除的长度
// 打开二进制文件
fstream file(filename, ios::in | ios::out | ios::binary);
if (!file) {
cerr << "Failed to open file: " << filename << endl;
return -1;
}
// 定位到需要删除的位置
file.seekp(-len_to_delete, ios::end);
// 截取文件内容
char* buf = new char[len_to_delete];
file.read(buf, len_to_delete);
// 重新定位到需要删除的位置
file.seekp(-len_to_delete, ios::end);
// 将截取后的内容写回到原文件中
file.write(buf, len_to_delete);
// 关闭文件并释放内存
file.close();
delete[] buf;
cout << "Successfully deleted " << len_to_delete << " bytes from file: " << filename << endl;
return 0;
}
```
需要注意的是,文件流在写入内容时会覆盖原文件中的内容,因此需要先将需要删除的内容截取出来再进行写入。另外,需要使用 seekp() 函数来定位到需要删除的位置。
相关推荐
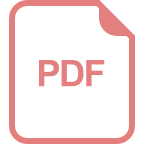
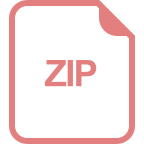
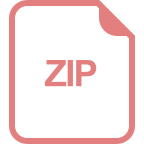














