C语言利用指针做二元一次方程
时间: 2023-06-13 19:06:29 浏览: 194
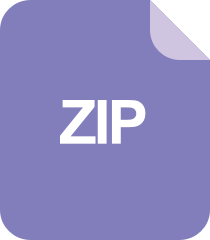
解二元一次方程.zip
可以利用指针来解二元一次方程组,具体步骤如下:
1. 定义二元一次方程组的系数矩阵和常数矩阵:
```c
float coef[2][2] = {{a1, b1}, {a2, b2}};
float cons[2] = {c1, c2};
```
2. 定义指向这两个矩阵的指针:
```c
float (*pcoef)[2] = coef;
float *pcons = cons;
```
3. 利用高斯-约旦消元法解出方程组的未知数:
```c
float x, y;
float k = *(pcoef[1] + 0) / *(pcoef[0] + 0);
*(pcoef[1] + 1) -= k * *(pcoef[0] + 1);
*(pcons + 1) -= k * *(pcons + 0);
y = *(pcons + 1) / *(pcoef[1] + 1);
x = (*(pcons + 0) - *(pcoef[0] + 1) * y) / *(pcoef[0] + 0);
```
在上述代码中,利用指针访问系数矩阵和常数矩阵的元素,并利用高斯-约旦消元法解出未知数x和y。
完整代码如下:
```c
#include <stdio.h>
int main() {
float a1, b1, c1, a2, b2, c2;
printf("Enter the coefficients of the first equation:\n");
scanf("%f%f%f", &a1, &b1, &c1);
printf("Enter the coefficients of the second equation:\n");
scanf("%f%f%f", &a2, &b2, &c2);
float coef[2][2] = {{a1, b1}, {a2, b2}};
float cons[2] = {c1, c2};
float (*pcoef)[2] = coef;
float *pcons = cons;
float x, y;
float k = *(pcoef[1] + 0) / *(pcoef[0] + 0);
*(pcoef[1] + 1) -= k * *(pcoef[0] + 1);
*(pcons + 1) -= k * *(pcons + 0);
y = *(pcons + 1) / *(pcoef[1] + 1);
x = (*(pcons + 0) - *(pcoef[0] + 1) * y) / *(pcoef[0] + 0);
printf("x: %f, y: %f\n", x, y);
return 0;
}
```
阅读全文
相关推荐
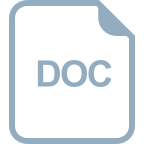
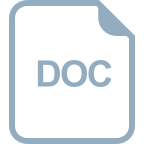
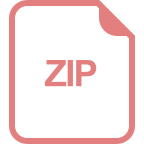
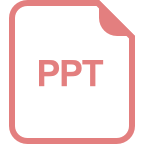
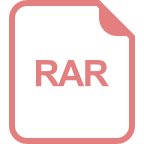
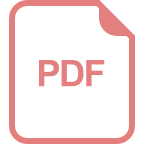
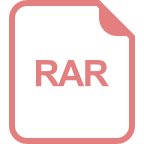
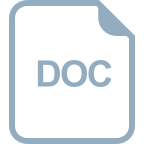
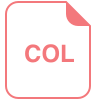


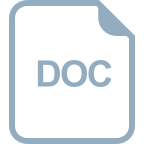
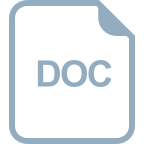
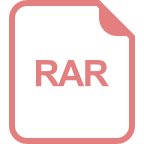