用单链表实现学生信息管理系统的设计与实现
时间: 2023-08-14 08:04:17 浏览: 41
好的,我可以为您讲解一下用单链表实现学生信息管理系统的设计与实现。
首先,我们需要定义一个学生的结构体,包括学号、姓名、性别、年龄等基本信息。如下所示:
```c
typedef struct Student {
int id; // 学号
char name[20]; // 姓名
char sex[10]; // 性别
int age; // 年龄
struct Student* next; // 指向下一个节点的指针
} Student;
```
接下来,我们需要定义一些基本的操作函数,用于管理学生信息,例如插入、删除、查询等操作。这里以插入操作为例:
```c
// 在链表头插入一个学生
Student* insert(Student* head, int id, char* name, char* sex, int age) {
Student* new_student = (Student*)malloc(sizeof(Student));
new_student->id = id;
strcpy(new_student->name, name);
strcpy(new_student->sex, sex);
new_student->age = age;
new_student->next = head;
head = new_student;
return head;
}
```
在这个函数中,我们首先创建了一个新的学生节点,然后将其基本信息填充好,并将其指针指向链表头,最后将链表头指向新节点。这样,我们就完成了一个学生信息的插入操作。
其他操作函数的实现方式与插入类似,可以根据需要进行修改和扩展。最后,我们可以通过遍历链表的方式输出所有的学生信息,如下所示:
```c
// 输出所有学生信息
void print_list(Student* head) {
printf("学号\t姓名\t性别\t年龄\n");
while (head) {
printf("%d\t%s\t%s\t%d\n", head->id, head->name, head->sex, head->age);
head = head->next;
}
}
```
这样,我们就完成了一个简单的学生信息管理系统的设计与实现。
相关推荐
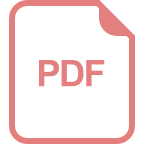
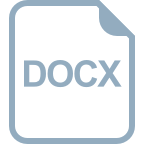
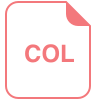
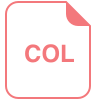
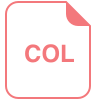











