请给出python实现粒子群优化算法的代码
时间: 2023-10-01 12:02:16 浏览: 98
以下是Python实现粒子群优化算法的示例代码:
```python
import random
import math
class Particle:
def __init__(self, x, y):
self.x = x
self.y = y
self.vx = 0
self.vy = 0
self.bestx = x
self.besty = y
self.bestscore = float('inf')
self.score = float('inf')
def update_velocity(self, bestx, besty, w, c1, c2):
self.vx = w * self.vx + c1 * random.random() * (self.bestx - self.x) + c2 * random.random() * (bestx - self.x)
self.vy = w * self.vy + c1 * random.random() * (self.besty - self.y) + c2 * random.random() * (besty - self.y)
def update_position(self):
self.x += self.vx
self.y += self.vy
def evaluate(self, func):
self.score = func(self.x, self.y)
if self.score < self.bestscore:
self.bestscore = self.score
self.bestx = self.x
self.besty = self.y
def particle_swarm(func, bounds, n_particles, n_iter, w, c1, c2):
particles = [Particle(random.uniform(bounds[0][0], bounds[1][0]), random.uniform(bounds[0][1], bounds[1][1])) for i in range(n_particles)]
bestscore = float('inf')
bestx = None
besty = None
for i in range(n_iter):
for particle in particles:
particle.evaluate(func)
if particle.score < bestscore:
bestscore = particle.score
bestx = particle.x
besty = particle.y
for particle in particles:
particle.update_velocity(bestx, besty, w, c1, c2)
particle.update_position()
particle.x = max(min(particle.x, bounds[1][0]), bounds[0][0])
particle.y = max(min(particle.y, bounds[1][1]), bounds[0][1])
return (bestx, besty, bestscore)
```
该代码定义了一个 `Particle` 类来表示每个粒子,包含了位置、速度、历史最优位置和历史最优得分等属性,以及更新速度、更新位置和评估得分等方法。
`particle_swarm` 函数实现了粒子群优化算法,接受函数、变量边界、粒子数、迭代次数、惯性权重、个体学习因子和群体学习因子等参数。在函数内部,先初始化了一组粒子,然后在每次迭代中,先评估每个粒子的得分,更新全局最优解,然后更新每个粒子的速度和位置,并确保它们在变量边界范围内。最后返回全局最优解的位置和得分。
阅读全文
相关推荐





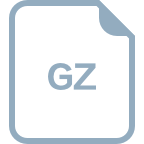










