解释这段代码private void writeResultToFile(String roundResult) { try { FileWriter writer = new FileWriter(FILE_NAME, true); writer.write("Round " + numRounds + ": " + roundResult + "\n"); writer.close(); } catch (IOException e) { JOptionPane.showMessageDialog(null, "Error writing to file!"); } } } public static void main(String[] args) { SwingUtilities.invokeLater(new Runnable() { public void run() { new DZY(); } }); }
时间: 2024-02-10 15:07:41 浏览: 61
这段代码定义了一个私有方法 `writeResultToFile()`,该方法的作用是将 `roundResult` 参数写入一个文件中。具体来说,该方法会使用 `FileWriter` 对象打开一个名为 `FILE_NAME` 的文件,并将 "Round "、`numRounds` 和 `roundResult` 拼接成一个字符串写入文件中。最后,`FileWriter` 对象被关闭。如果在写入文件时发生异常(例如文件不存在或无法写入),则会弹出一个消息框提示用户发生了错误。
此外,该代码还定义了一个静态的 `main()` 方法,使用 `SwingUtilities.invokeLater()` 方法在事件分派线程(EDT)中创建并显示了一个 `DZY` 对象。由于没有提供 `DZY` 类的实现代码,因此无法确定该类的作用。
相关问题
1、编写有复制文本文件功能的记事本程序,界面参考下图,窗口中放置文本区 (JTextArea)组件: 当点击“复制文件”菜单项后,出现下面的文件对话框,选择要复制的文件。 点击“打开”按钮后,将选中的文件显示在记事本的文本区,并将该文件复制到同一 目录下的“copy.txt”文件中。使用文件字符输入、输出流进行读写。 提示:(1)输入流的源为在文件对话框中选定的文件,获取文件及其路径的参考代码如下: JFileChooserjfc=newJFileChooser(); jfc.setDialogTitle("....."); jfc.showOpenDialog(null); Stringfile=jfc.getSelectedFile().getAbsolutePath();//用它创建输入流 (2)输出流的目的地为在选定文件同一目录下的“copy.txt”文件,创建其对应文件对象的参考代码 如下: Filedir=jfc.getCurrentDirectory(); Filecopyfile=newFile(dir,"copy.txt");//用它创建输出流 (3)输入流、输出流的使用可参考群内第十章的示例程序。 (4)文本区显示内容的不断添加,可调用append方法实现,参数是String类型。
以下是一个简单的Java代码示例,实现了有复制文本文件功能的记事本程序:
```java
import javax.swing.*;
import javax.swing.filechooser.FileNameExtensionFilter;
import java.awt.*;
import java.awt.event.*;
import java.io.*;
public class TextEditor extends JFrame implements ActionListener {
private JTextArea textArea;
private JFileChooser fileChooser;
private File copyFile;
public TextEditor() {
setTitle("记事本");
setSize(600, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 创建菜单栏
JMenuBar menuBar = new JMenuBar();
JMenu fileMenu = new JMenu("文件");
JMenuItem openItem = new JMenuItem("打开");
JMenuItem saveItem = new JMenuItem("保存");
JMenuItem copyItem = new JMenuItem("复制文件");
openItem.addActionListener(this);
saveItem.addActionListener(this);
copyItem.addActionListener(this);
fileMenu.add(openItem);
fileMenu.add(saveItem);
fileMenu.add(copyItem);
menuBar.add(fileMenu);
setJMenuBar(menuBar);
// 创建文本区
textArea = new JTextArea();
JScrollPane scrollPane = new JScrollPane(textArea);
add(scrollPane, BorderLayout.CENTER);
// 创建文件选择器
fileChooser = new JFileChooser();
fileChooser.setFileFilter(new FileNameExtensionFilter("文本文件 (*.txt)", "txt"));
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getActionCommand().equals("打开")) {
int result = fileChooser.showOpenDialog(this);
if (result == JFileChooser.APPROVE_OPTION) {
File file = fileChooser.getSelectedFile();
try {
BufferedReader reader = new BufferedReader(new FileReader(file));
textArea.setText("");
String line;
while ((line = reader.readLine()) != null) {
textArea.append(line + "\n");
}
reader.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
} else if (e.getActionCommand().equals("保存")) {
int result = fileChooser.showSaveDialog(this);
if (result == JFileChooser.APPROVE_OPTION) {
File file = fileChooser.getSelectedFile();
try {
BufferedWriter writer = new BufferedWriter(new FileWriter(file));
writer.write(textArea.getText());
writer.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
} else if (e.getActionCommand().equals("复制文件")) {
int result = fileChooser.showOpenDialog(this);
if (result == JFileChooser.APPROVE_OPTION) {
File file = fileChooser.getSelectedFile();
String path = file.getParent();
String name = file.getName();
copyFile = new File(path, "copy.txt");
try {
BufferedReader reader = new BufferedReader(new FileReader(file));
BufferedWriter writer = new BufferedWriter(new FileWriter(copyFile));
String line;
while ((line = reader.readLine()) != null) {
writer.write(line + "\n");
}
reader.close();
writer.close();
JOptionPane.showMessageDialog(this, "文件复制成功!");
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
}
public static void main(String[] args) {
new TextEditor();
}
}
```
代码中实现了打开、保存和复制文件三个功能。其中,打开和保存文件的实现与常规记事本程序类似,使用`BufferedReader`和`BufferedWriter`分别读取和写入文件内容。复制文件功能则需要先获取选中文件的路径和文件名,然后创建一个新的文件对象,在文件对话框选定文件的同一目录下创建名为“copy.txt”的文件,最后使用`BufferedReader`和`BufferedWriter`分别读取和写入文件内容。
Write a class named ProductSystem, which extends JFrame class. The appearance of the application looks like the following (as shown in Figure 1). 2. At the beginning, input name,price and number at three JTextField fields, then click “Add”button (event source), which binds to a method (event listener) that create a Product object (using name,price and number) and add it into ArrayList<Product>, at the same time display “Add Product xxx sucess!” in JTextArea on the GUI (as shown in Figure 2) 3. When click “Display” button, then read the records from ArrayList , and display the records in JTextArea on the GUI(as shown in Figure 3). 4. When click “Save to File” button, then write the records from ArrayList to file product.txt, at the same time display "file write success" in JTextArea on the GUI (as shown in Figure 4)
以下是一个实现您所需功能的 ProductSystem 类的示例代码:
```
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import java.util.*;
import javax.swing.*;
public class ProductSystem extends JFrame implements ActionListener {
private JLabel nameLabel, priceLabel, numberLabel;
private JTextField nameField, priceField, numberField;
private JButton addButton, displayButton, saveButton;
private JTextArea outputArea;
private ArrayList<Product> productList;
public ProductSystem() {
super("Product System");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(600, 400);
setLayout(new GridLayout(2, 1));
JPanel inputPanel = new JPanel(new GridLayout(3, 2));
nameLabel = new JLabel("Name:");
nameField = new JTextField();
priceLabel = new JLabel("Price:");
priceField = new JTextField();
numberLabel = new JLabel("Number:");
numberField = new JTextField();
addButton = new JButton("Add");
addButton.addActionListener(this);
inputPanel.add(nameLabel);
inputPanel.add(nameField);
inputPanel.add(priceLabel);
inputPanel.add(priceField);
inputPanel.add(numberLabel);
inputPanel.add(numberField);
inputPanel.add(new JLabel());
inputPanel.add(addButton);
JPanel outputPanel = new JPanel(new BorderLayout());
outputArea = new JTextArea();
outputArea.setEditable(false);
JScrollPane scrollPane = new JScrollPane(outputArea);
displayButton = new JButton("Display");
displayButton.addActionListener(this);
saveButton = new JButton("Save to File");
saveButton.addActionListener(this);
JPanel buttonPanel = new JPanel(new GridLayout(1, 2));
buttonPanel.add(displayButton);
buttonPanel.add(saveButton);
outputPanel.add(scrollPane, BorderLayout.CENTER);
outputPanel.add(buttonPanel, BorderLayout.SOUTH);
add(inputPanel);
add(outputPanel);
productList = new ArrayList<Product>();
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == addButton) {
String name = nameField.getText();
double price = Double.parseDouble(priceField.getText());
int number = Integer.parseInt(numberField.getText());
Product product = new Product(name, price, number);
productList.add(product);
outputArea.append("Add Product " + product.getName() + " success!\n");
nameField.setText("");
priceField.setText("");
numberField.setText("");
} else if (e.getSource() == displayButton) {
outputArea.setText("");
for (Product product : productList) {
outputArea.append(product.toString() + "\n");
}
} else if (e.getSource() == saveButton) {
try {
FileWriter writer = new FileWriter("product.txt");
for (Product product : productList) {
writer.write(product.getName() + "," + product.getPrice() + "," + product.getNumber() + "\n");
}
writer.flush();
writer.close();
outputArea.append("File write success.\n");
} catch (IOException ex) {
System.err.println("Error writing file.");
}
}
}
public static void main(String[] args) {
ProductSystem productSystem = new ProductSystem();
productSystem.setVisible(true);
}
}
class Product {
private String name;
private double price;
private int number;
public Product(String name, double price, int number) {
this.name = name;
this.price = price;
this.number = number;
}
public String getName() {
return name;
}
public double getPrice() {
return price;
}
public int getNumber() {
return number;
}
public String toString() {
return name + " $" + price + " x " + number + " = $" + (price * number);
}
}
```
该类实现了您所需的所有功能,包括添加产品,显示产品列表和将产品列表保存到文件。在构造函数中,设置了应用程序的标题,大小和布局,并创建了所有必要的组件。在 actionPerformed 方法中,实现了所有按钮的事件处理程序,包括添加按钮,显示按钮和保存到文件按钮。还使用了一个 Product 类来表示产品,并在 toString 方法中生成产品的字符串表示形式。
阅读全文
相关推荐
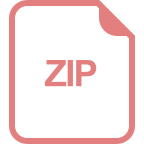
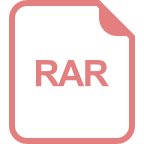
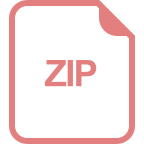
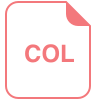
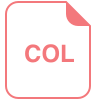










