如何将注意力机制Squeeze-and-Attention模块嵌入自己的pspnet网络,请基于pytorch网络给出代码,并附中文注释
时间: 2024-03-01 16:49:22 浏览: 87
好的,我来为您解答这个问题。
在PyTorch中,可以通过自定义一个Squeeze-and-Attention模块类来实现其在PSPNet网络中的嵌入。以下是一个示例代码,其中包括了一个PSPNet网络和一个Squeeze-and-Attention模块的嵌入。
```python
import torch
import torch.nn as nn
class SqueezeAndAttention(nn.Module):
def __init__(self, in_channels, reduction_ratio=16):
super(SqueezeAndAttention, self).__init__()
# 定义Squeeze操作,将输入的特征图压缩为一个全局向量
self.squeeze = nn.AdaptiveAvgPool2d(1)
# 定义Excitation操作,对全局向量进行特征重要性的学习
self.excitation = nn.Sequential(
nn.Linear(in_channels, in_channels // reduction_ratio),
nn.ReLU(inplace=True),
nn.Linear(in_channels // reduction_ratio, in_channels),
nn.Sigmoid()
)
def forward(self, x):
# 对输入的特征图进行Squeeze操作
y = self.squeeze(x)
# 将Squeeze操作后的特征图转换为一维向量
y = y.view(y.size(0), -1)
# 对Squeeze操作后的特征向量进行Excitation操作,得到特征重要性向量
y = self.excitation(y)
# 将特征重要性向量应用到输入的特征图上,得到加权后的特征图
y = y.view(y.size(0), y.size(1), 1, 1)
y = x * y.expand_as(x)
return y
class PSPNet(nn.Module):
def __init__(self, num_classes):
super(PSPNet, self).__init__()
# 定义PSPNet网络的各个模块
self.features = nn.Sequential(
nn.Conv2d(3, 64, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
# 加入一个Squeeze-and-Attention模块
SqueezeAndAttention(64),
nn.Conv2d(64, 128, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(128),
nn.ReLU(inplace=True),
nn.Conv2d(128, 256, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(256, 512, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(512),
nn.ReLU(inplace=True),
# 加入一个Squeeze-and-Attention模块
SqueezeAndAttention(512),
nn.Conv2d(512, 1024, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(1024),
nn.ReLU(inplace=True),
nn.Conv2d(1024, 1024, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(1024),
nn.ReLU(inplace=True)
)
# 定义PSPNet的Pyramid Pooling模块
self.pyramid_pooling = nn.Sequential(
nn.AdaptiveAvgPool2d(1),
nn.Conv2d(1024, 256, kernel_size=1, stride=1),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(256, 256, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(256, num_classes, kernel_size=1, stride=1)
)
def forward(self, x):
# 对输入的图像进行特征提取
x = self.features(x)
# 对提取的特征图进行Pyramid Pooling
x = self.pyramid_pooling(x)
# 对Pyramid Pooling后的特征图进行上采样,得到最终的分割结果
x = nn.functional.interpolate(x, scale_factor=8, mode='bilinear', align_corners=True)
return x
# 实例化一个PSPNet对象
num_classes = 21
net = PSPNet(num_classes)
# 定义一个输入数据,形状为(batch_size, channels, H, W)
inputs = torch.randn(16, 3, 224, 224)
# 前向计算,得到PSPNet的分割结果
outputs = net(inputs)
print(outputs.shape) # 输出形状为(batch_size, num_classes, H/8, W/8)
```
在这个示例代码中,我们首先定义了一个Squeeze-and-Attention模块类,其中包含Squeeze操作和Excitation操作。在Squeeze操作中,我们使用AdaptiveAvgPool2d将输入的特征图压缩为一个全局向量。在Excitation操作中,我们使用线性变换和sigmoid函数对全局向量进行特征重要性的学习。在forward方法中,我们将Squeeze操作后的特征向量应用到输入的特征图上,得到加权后的特征图。
在实例化PSPNet对象后,我们可以将输入数据传递给net对象,进行前向计算。最终,我们可以得到PSPNet的分割结果的形状,并将其打印出来。
希望这个示例代码能够帮助到您,如果您还有其他问题,请随时提问!
阅读全文
相关推荐
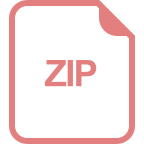
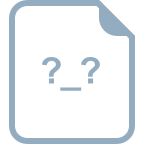
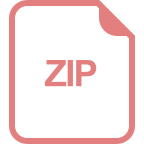

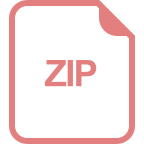
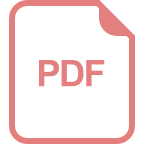
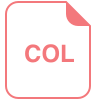











