编写4个子函数,分别实现:统计大写字母数量、统计小写字母数量、统计数字数量、统计空格数量。
时间: 2023-09-22 20:05:46 浏览: 74
### 回答1:
好的,以下是实现这四个子函数的代码:
```python
def count_uppercase(s):
return sum(1 for c in s if c.isupper())
def count_lowercase(s):
return sum(1 for c in s if c.islower())
def count_digits(s):
return sum(1 for c in s if c.isdigit())
def count_spaces(s):
return sum(1 for c in s if c.isspace())
```
这些函数都接受一个字符串作为参数,并返回相应的计数。例如,`count_uppercase("Hello, World!")` 将返回 2,因为该字符串中有两个大写字母。
### 回答2:
编写四个子函数,分别实现统计大写字母数量、统计小写字母数量、统计数字数量和统计空格数量。
子函数一:统计大写字母数量
```python
def count_uppercase(text):
count = 0
for char in text:
if char.isupper():
count += 1
return count
```
子函数二:统计小写字母数量
```python
def count_lowercase(text):
count = 0
for char in text:
if char.islower():
count += 1
return count
```
子函数三:统计数字数量
```python
def count_digits(text):
count = 0
for char in text:
if char.isdigit():
count += 1
return count
```
子函数四:统计空格数量
```python
def count_spaces(text):
count = 0
for char in text:
if char.isspace():
count += 1
return count
```
以上分别为四个子函数的实现。可以将需要统计的文本作为参数传入这些函数,返回对应的统计结果。例如,假设要统计文本"Hello World! 123"中的大写字母数量、小写字母数量、数字数量和空格数量,可以如下调用:
```python
text = "Hello World! 123"
uppercase_count = count_uppercase(text)
lowercase_count = count_lowercase(text)
digits_count = count_digits(text)
spaces_count = count_spaces(text)
print("大写字母数量:", uppercase_count)
print("小写字母数量:", lowercase_count)
print("数字数量:", digits_count)
print("空格数量:", spaces_count)
```
运行结果为:
```
大写字母数量: 2
小写字母数量: 8
数字数量: 3
空格数量: 2
```
### 回答3:
编写4个子函数,实现统计大写字母数量、统计小写字母数量、统计数字数量、统计空格数量的功能。
1. 统计大写字母数量:
```python
def count_uppercase(text):
count = 0
for char in text:
if char.isupper():
count += 1
return count
```
2. 统计小写字母数量:
```python
def count_lowercase(text):
count = 0
for char in text:
if char.islower():
count += 1
return count
```
3. 统计数字数量:
```python
def count_digits(text):
count = 0
for char in text:
if char.isdigit():
count += 1
return count
```
4. 统计空格数量:
```python
def count_spaces(text):
count = 0
for char in text:
if char.isspace():
count += 1
return count
```
以上是四个独立的子函数,你可以将需要统计的文本作为参数传递给这些函数,然后得到相应的统计结果。
相关推荐
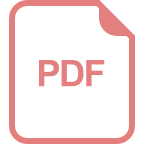
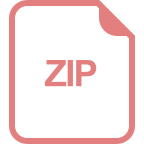
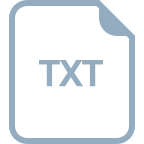














