// Create a controller late final AnimationController _controller = AnimationController( duration: const Duration(seconds: 2), vsync: this, )..repeat(reverse: false); // Create an animation with value of type "double" late final Animation<double> _animation = CurvedAnimation( parent: _controller, curve: Curves.linear, );
时间: 2024-04-01 13:36:52 浏览: 94
这段代码创建了一个 `AnimationController` 对象 `_controller` 和一个 `CurvedAnimation` 对象 `_animation`。`AnimationController` 用于控制动画的播放,而 `CurvedAnimation` 则用于定义动画的曲线。
`AnimationController` 的参数中:
- `duration` 指定了动画的持续时间,这里设置为 2 秒钟。
- `vsync` 参数指定了垂直同步对象,通常应该设置为当前 `State` 中的 `TickerProviderStateMixin`。
`AnimationController` 对象的 `repeat` 方法用于设置动画重复播放,并可以指定是否反向播放动画。这里我们设置 `reverse` 参数为 `false`,表示不反向播放动画。
`CurvedAnimation` 的参数中:
- `parent` 参数指定了它所依赖的 `AnimationController` 对象,这里设置为 `_controller`。
- `curve` 参数指定了动画的曲线,这里我们使用了 `Curves.linear`,表示匀速变化。
使用这两个对象,我们可以创建一个 `Tween` 对象来定义动画的开始和结束状态。例如,下面的代码创建了一个 `Tween<double>`,将动画的值从 0.0 逐渐变化到 1.0:
```dart
final Tween<double> tween = Tween<double>(begin: 0.0, end: 1.0);
```
我们可以使用这个 `tween` 对象来创建一个 `AnimatedBuilder`,在其中构建动画的具体效果。例如,下面的代码在窗口中显示一个红色的方块,它的宽度会随着动画的进度而变化:
```dart
AnimatedBuilder(
animation: _animation,
builder: (BuildContext context, Widget child) {
return Container(
width: tween.evaluate(_animation) * 200,
height: 100,
color: Colors.red,
);
},
);
```
在这个示例中,我们将 `AnimatedBuilder` 的 `animation` 参数设置为 `_animation`,表示它将根据 `_animation` 的值来更新动画。在 `builder` 回调函数中,我们根据动画的进度计算出当前的宽度值,然后用它来设置方块的宽度。由于动画的进度值在 0.0 到 1.0 之间变化,我们将其乘以 200,以使方块的宽度在 0 到 200 之间变化。
阅读全文
相关推荐
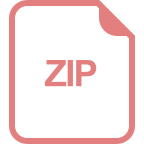
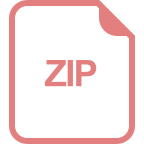
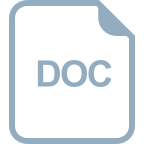
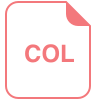
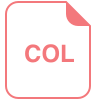
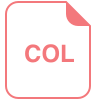
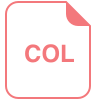
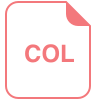
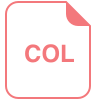
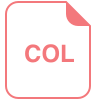
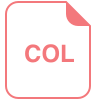
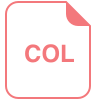
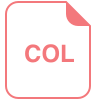
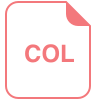
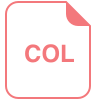
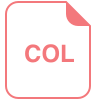
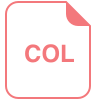