Flutter动画入门:基础动画与动画控制器的使用
发布时间: 2023-12-20 08:03:07 阅读量: 42 订阅数: 23 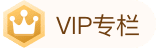
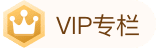
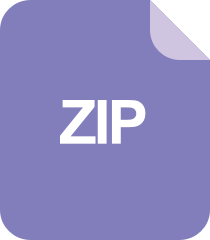
Flutter动画基础教程
# 第一章:介绍Flutter动画
## 1.1 什么是Flutter动画
在Flutter中,动画是指一系列有规律的图像变化,通过连续的图像帧展示,给用户创造出一种运动的错觉。Flutter动画通过逐帧动画和补间动画方式实现。
逐帧动画是指将一系列静态图片以一定的速度连续播放,形成动画效果。而补间动画是指定义起始状态和结束状态,然后由系统自行计算并展示中间过渡状态,从而实现动画效果。
## 1.2 Flutter动画的重要性
在移动应用开发中,动画是提升用户体验的重要手段之一。良好的动画效果可以增强用户对应用的好感度,使应用更加生动有趣。Flutter强大的动画库使得开发者可以轻松实现各种炫酷的动画效果,为应用增色不少。
## 第二章:基础动画
### 2.1 使用AnimatedContainer进行基础动画
在Flutter中,可以使用`AnimatedContainer` widget轻松实现基础动画。`AnimatedContainer`可以在一定时间内从一个样式过渡到另一个样式,比如颜色、大小、边框等的过渡。
```python
class BasicAnimationPage extends StatefulWidget {
@override
_BasicAnimationPageState createState() => _BasicAnimationPageState();
}
class _BasicAnimationPageState extends State<BasicAnimationPage> {
bool _isChanged = false;
// 点击按钮时切换动画状态
void _toggleColor() {
setState(() {
_isChanged = !_isChanged;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('基础动画 - AnimatedContainer'),
),
body: Center(
child: AnimatedContainer(
duration: Duration(seconds: 1),
width: _isChanged ? 200.0 : 100.0,
height: _isChanged ? 100.0 : 200.0,
color: _isChanged ? Colors.blue : Colors.red,
curve: Curves.easeInOut, // 添加曲线动画
),
),
floatingActionButton: FloatingActionButton(
onPressed: _toggleColor,
child: Icon(Icons.play_arrow),
),
);
}
}
```
**代码说明:**
- 首先定义一个`_isChanged`变量来控制是否执行动画效果。
- 使用`AnimatedContainer`包裹需要执行动画的部件,设置`duration`属性来定义动画时长,在点击按钮时通过设置宽高和颜色的变化来展现动画效果。
- `curve`属性可以添加曲线动画,让动画更加自然流畅。
- 点击悬浮按钮时,调用`_toggleColor`方法切换动画状态。
**运行效果:**
点击悬浮按钮时,可以看到容器的宽高和颜色会平滑过渡,呈现出动画效果。
### 3. 第三章:常见动画效果
在本章中,我们将介绍如何实现常见的动画效果,包括渐变动画、旋转动画和缩放动画。通过学习这些内容,您将能够掌握在Flutter中实现常见动画效果的方法。
#### 3.1 实现渐变动画
渐变动画是一种常见的动画效果,可以让UI元素的颜色在一段时间内平滑过渡。在Flutter中,我们可以使用`AnimatedContainer`和`DecorationTween`来实现颜色渐变动画。以下是一个简单的示例代码:
```dart
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: GradientAnimationDemo(),
);
}
}
class GradientAnimationDemo extends StatefulWidget {
@override
_GradientAnimationDemoState createState() => _GradientAnimationDemoState();
}
class _GradientAnimationDemoState extends State<GradientAnimationDemo>
with SingleTickerProviderStateMixin {
late AnimationController _controller;
late Animation<Decoration> _animation;
@override
void initState() {
super.initState();
_controller = AnimationController(
duration: Duration(seconds: 2),
vsync: this,
);
_animation = DecorationTween(
begin: BoxDecoration(
gradient: LinearGradient(
colors: [Colors.blue, Colors.green],
),
),
end: BoxDecoration(
gradient: LinearGradient(
colors: [Colors.red, Colors.yellow],
),
),
).animate(_controller);
_controller.repeat(reverse: true);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Gradient Animation Demo'),
),
body: Center(
child: AnimatedBuilder(
animation: _animation,
builder: (context, child) {
return Container(
width: 200,
height: 200,
decoration: _animation.value,
);
},
),
),
);
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
}
```
**代码说明:**
- 我们使用`AnimatedBuilder`来构建一个动画渐变的Container,通过改变`Decoration`来实现颜色渐变效果。
- 在`AnimationController`中设置动画的持续时间,并使用`DecorationTween`来定义颜色渐变的起始和结束状态。
- 最后,我们将动画控制器设置为循环播放,并在状态销毁时释放资源。
**运行结果:**
当您运行此示例时,您将看到一个方形容器在蓝色和绿色之间渐变到红色和黄色,然后再从红色和黄色渐变到蓝色和绿色的动画效果。
#### 3.2 实现旋转动画
旋转动画可以让UI元素围绕自身中心旋转,为界面增添活力。在Flutter中,我们可以使用`RotationTransition`来实现旋转动画。以下是一个简单的旋转动画示例代码:
```dart
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: RotationAnimationDemo(),
);
}
}
class RotationAnimationDemo extends StatefulWidget {
@override
_RotationAnimationDemoState createState() => _RotationAnimationDemoState();
}
class _RotationAnimationDemoState extends State<RotationAnimationDemo>
with SingleTickerProviderStateMixin {
late AnimationController _controller;
late Animation<double> _animation;
@override
void initState() {
super.initState();
_controller = AnimationController(
duration: Duration(seconds: 2),
vsync: this,
);
_animation = Tween(begin: 0.0, end: 2 * 3.14).animate(_controller)
..addListener(() {
setState(() {});
});
_controller.repeat(reverse: true);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Rotation Animation Demo'),
),
body: Center(
child: Tr
```
0
0
相关推荐






