定义一个函数,函数功能是求长方体的体积(给出默认参数值长4,),在主函数中分别使用默认参数值、使用新的实参给出长宽高两次调用该函数。
时间: 2023-04-29 22:03:02 浏览: 150
以下是定义函数的代码:
```python
def volume(length=4, width=4, height=4):
return length * width * height
```
在主函数中,我们可以分别使用默认参数值和新的实参来调用该函数:
```python
# 使用默认参数值调用函数
print(volume()) # 输出: 64
# 使用新的实参调用函数
print(volume(2, 3, 4)) # 输出: 24
```
第一次调用函数时,我们没有给出任何实参,因此函数使用了默认参数值,即长、宽、高均为4,计算出的体积为64。
第二次调用函数时,我们给出了新的实参,即长为2、宽为3、高为4,函数使用这些实参计算出的体积为24。
相关问题
用c++请定义一个名为RECT的类,实现计算一个长方体体积的功能; 类中要包含默认构造函数,实现默认情况下长方体的长(a)、宽(b)和高()三个参 数的值都为0; 类中要包含带参数的构造函数,实现通过参数对长方体的长(a)、宽(b)和高(h三个数进行初始化赋值;。 类中要包含成员函数display(),实现计算长方体体积,并将体积值输出到屏幕上。 主函数中定义两个RECT类的对象rect1、rect2,计算长(a)、宽((b)、高()分别为 2、3、10和4、5、20这两个长方体的体积值。
以下是实现题目要求的代码:
```c++
#include <iostream>
using namespace std;
class RECT {
private:
double length, width, height;
public:
RECT() {
length = 0;
width = 0;
height = 0;
}
RECT(double l, double w, double h) {
length = l;
width = w;
height = h;
}
void display() {
double volume = length * width * height;
cout << "Length: " << length << endl;
cout << "Width: " << width << endl;
cout << "Height: " << height << endl;
cout << "Volume: " << volume << endl;
}
};
int main() {
RECT rect1(2, 3, 10);
RECT rect2(4, 5, 20);
rect1.display();
rect2.display();
return 0;
}
```
在上述代码中,RECT 类包含了两个构造函数,一个是默认构造函数,一个是带参数的构造函数。display() 函数用于计算长方体体积并输出结果。在主函数中,定义了两个 RECT 类的对象 rect1 和 rect2,并对其成员变量进行初始化。最后调用 display() 函数输出计算结果。
设计一个长方体类,其长、宽和高属性默认为1,其成员函数计算长方体的体积。为该类的长、宽和高设置set和get函数。Set函数应验证长、宽和高均为8-50之间的浮点数。 要求包括:(1)定义无参的构造函数、析构函数、(2)在主函数中输入长方体长、宽、高(实参值),定义长方体类的对象,调用计算长方体类的体积的函数。
以下是用 C++ 语言描述的长方体类:
```
class Rectangle
{
private:
float length;
float width;
float height;
public:
// 构造函数,初始化长方体的长、宽、高
Rectangle(float l=1, float w=1, float h=1)
{
setLength(l);
setWidth(w);
setHeight(h);
}
// 析构函数
~Rectangle() {}
// set 函数,用于设置长、宽、高的值
void setLength(float l)
{
if (l > 8 && l < 50)
length = l;
else
length = 1;
}
void setWidth(float w)
{
if (w > 8 && w < 50)
width = w;
else
width = 1;
}
void setHeight(float h)
{
if (h > 8 && h < 50)
height = h;
else
height = 1;
}
// get 函数,用于获取长、宽、高的值
float getLength() { return length; }
float getWidth() { return width; }
float getHeight() { return height; }
// 计算长方体的体积
float getVolume() { return length * width * height; }
};
```
在主函数中,你可以这样调用:
```
int main()
{
// 定义一个长方体类的对象,并初始化
Rectangle rect(10, 20, 30);
// 调用 getVolume 函数,计算长方体的体积
float volume = rect.getVolume();
cout << "长方体的体积:" << volume << endl;
return 0;
}
```
阅读全文
相关推荐
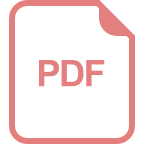
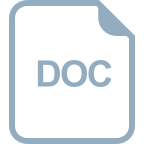
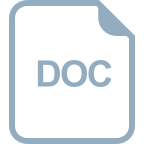
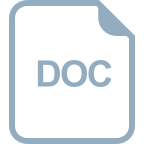



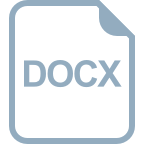
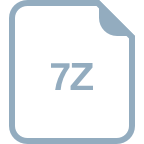
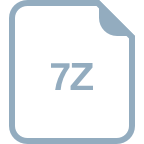
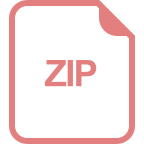