制作一个登陆时输入账号以区分读者和管理员,并且管理员可以增加、修改、删除图书。读者能够查询、借阅、归还、显示个人借阅历史及所借图书状态的代码
时间: 2023-07-10 16:24:41 浏览: 51
好的,以下是一个简单的Python图书馆管理系统的代码示例,包含了登陆时输入账号以区分读者和管理员、管理员可以增加、修改、删除图书、读者能够查询、借阅、归还、显示个人借阅历史及所借图书状态的功能:
```python
class Book:
def __init__(self, title, author, status):
self.title = title
self.author = author
self.status = status
class Library:
books = []
@classmethod
def add_book(cls, book):
cls.books.append(book)
@classmethod
def remove_book(cls, book):
cls.books.remove(book)
@classmethod
def update_book(cls, book, title=None, author=None, status=None):
if title:
book.title = title
if author:
book.author = author
if status:
book.status = status
@classmethod
def search_books(cls, title=None, author=None, status=None):
results = []
for book in cls.books:
if title and title.lower() not in book.title.lower():
continue
if author and author.lower() not in book.author.lower():
continue
if status and status.lower() != book.status.lower():
continue
results.append(book)
return results
class User:
def __init__(self, username, password, is_admin=False):
self.username = username
self.password = password
self.is_admin = is_admin
self.borrowed_books = []
def borrow_book(self, book):
if book.status != 'available':
return False
book.status = 'borrowed'
self.borrowed_books.append(book)
return True
def return_book(self, book):
if book not in self.borrowed_books:
return False
book.status = 'available'
self.borrowed_books.remove(book)
return True
def get_borrowed_books(self):
return self.borrowed_books
class LibrarySystem:
users = []
@classmethod
def register_user(cls, username, password, is_admin=False):
user = User(username, password, is_admin)
cls.users.append(user)
return user
@classmethod
def login(cls, username, password):
for user in cls.users:
if user.username == username and user.password == password:
return user
return None
@classmethod
def is_admin(cls, user):
return user.is_admin
@classmethod
def add_book(cls, book):
Library.add_book(book)
@classmethod
def remove_book(cls, book):
Library.remove_book(book)
@classmethod
def update_book(cls, book, title=None, author=None, status=None):
Library.update_book(book, title, author, status)
@classmethod
def search_books(cls, title=None, author=None, status=None):
return Library.search_books(title, author, status)
if __name__ == '__main__':
user1 = LibrarySystem.register_user('user1', 'password1')
user2 = LibrarySystem.register_user('user2', 'password2')
admin = LibrarySystem.register_user('admin', 'adminpassword', True)
book1 = Book('Book 1', 'Author 1', 'available')
book2 = Book('Book 2', 'Author 2', 'available')
book3 = Book('Book 3', 'Author 3', 'borrowed')
LibrarySystem.add_book(book1)
LibrarySystem.add_book(book2)
LibrarySystem.add_book(book3)
user = LibrarySystem.login('user1', 'password1')
if user:
print('Welcome,', user.username)
books = LibrarySystem.search_books()
print('Available books:')
for book in books:
if book.status == 'available':
print(book.title)
book = books[0]
if user.borrow_book(book):
print('Successfully borrowed', book.title)
else:
print('Failed to borrow', book.title)
borrowed_books = user.get_borrowed_books()
print('Borrowed books:')
for book in borrowed_books:
print(book.title, book.status)
if user.return_book(book):
print('Successfully returned', book.title)
else:
print('Failed to return', book.title)
else:
print('Login failed')
user = LibrarySystem.login('admin', 'adminpassword')
if user and LibrarySystem.is_admin(user):
print('Welcome,', user.username)
book = books[1]
LibrarySystem.update_book(book, title='New Book 2')
LibrarySystem.remove_book(book)
new_book = Book('New Book', 'Author', 'available')
LibrarySystem.add_book(new_book)
books = LibrarySystem.search_books()
print('All books:')
for book in books:
print(book.title, book.author, book.status)
else:
print('Login failed')
```
该程序使用了面向对象的编程方法,包含了Book、Library、User、LibrarySystem四个类。其中,Book类表示图书,包含了书名、作者和状态(可用、已借出等);Library类表示图书馆,包含了增加、删除、修改和查询图书的功能;User类表示用户,包含了借阅、归还和获取个人借阅历史记录的功能;LibrarySystem类是整个系统的接口,包含了用户注册、登陆和管理员操作的功能。
在该程序中,用户可以通过输入用户名和密码进行登陆,如果是管理员账号则可以进行图书的增加、删除和修改,如果是普通读者则可以进行图书的查询、借阅和归还。同时,用户可以获取自己借阅的图书列表和个人借阅历史记录。
相关推荐
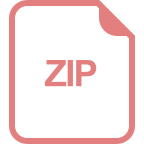
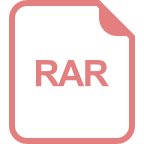
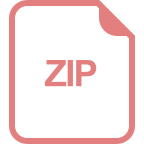














