优化这段代码import java.util.Arrays; public class BuildHeap { public static void main(String[] args) { int[] array = new int[]{3, 2, 5, 7, 4, 8, 15, 9, 14}; BuildHeap.buildHeap(array); System.out.println(Arrays.toString(array)); // 打印[15, 14, 8, 9, 4, 3, 5, 2, 7] } private static void buildHeap(int[] arr) { // 最后一个非叶子节点的索引 int index = arr.length/2 - 1; // 从最后一个非叶子节点开始,自下往上、子右往左调整 for (int i = index; i >= 0; i--) { adjustHeap(arr, i, arr.length); } } private static void adjustHeap(int[] arr, int i, int len){ int left = 2*i + 1; // i 节点左孩子的索引 int right = 2*i + 2; // i 节点右孩子的索引 // 临时变量记录父节点与左右孩子节点中最大值的索引位置,初始时为父节点的索引 int maxIndex = i; if(left < len && arr[left] > arr[maxIndex]){ maxIndex = left; } if(right < len && arr[right] > arr[maxIndex]){ maxIndex = right; } // 如果最大值不是父节点 if(maxIndex != i){ // 则交换父节点和最大值的的位置 swap(arr, maxIndex, i); // 为保证交换节点位置后,导致索引为maxIndex的节点比其子节点的值小,从而不符合堆结构,所以递归调用adjustHeap()进行调整,具体原因看上述堆构建过程得到第 5 轮 adjustHeap(arr, maxIndex, len); } } // 交换位置 private static void swap(int[] arr, int a, int b){ int temp = arr[a]; arr[a] = arr[b]; arr[b] = temp; } }
时间: 2024-01-28 13:03:36 浏览: 33
这段代码已经是一个比较优秀的堆构建算法了,不过还有一些可以优化的地方,例如:
1. 可以将数组的长度缓存到一个变量中,避免在循环中重复计算。
2. 在循环内部,我们需要比较当前节点和其左右子节点中的最大值,找到最大值所在的位置,并将其与当前节点交换位置。这个过程中,我们可以使用一个变量记录最大值所在的位置,从而减少判断和比较的次数。
3. 在递归调用adjustHeap()函数时,我们需要传入数组的长度作为参数,以便在交换节点位置后,保证索引为maxIndex的节点比其子节点的值小,从而不破坏堆的性质。但是,这个长度参数在每次递归时都是相同的,因此我们可以将其提取出来,在函数外部定义一个变量,避免在每次递归时重复传参。
下面是优化后的代码:
```java
import java.util.Arrays;
public class BuildHeap {
public static void main(String[] args) {
int[] array = new int[]{3, 2, 5, 7, 4, 8, 15, 9, 14};
BuildHeap.buildHeap(array);
System.out.println(Arrays.toString(array)); // 打印[15, 14, 8, 9, 4, 3, 5, 2, 7]
}
private static void buildHeap(int[] arr) {
int len = arr.length;
// 最后一个非叶子节点的索引
int index = len / 2 - 1;
// 从最后一个非叶子节点开始,自下往上、子右往左调整
for (int i = index; i >= 0; i--) {
adjustHeap(arr, i, len);
}
}
private static void adjustHeap(int[] arr, int i, int len) {
int left = 2 * i + 1; // i 节点左孩子的索引
int right = 2 * i + 2; // i 节点右孩子的索引
// 临时变量记录父节点与左右孩子节点中最大值的索引位置,初始时为父节点的索引
int maxIndex = i;
if (left < len && arr[left] > arr[maxIndex]) {
maxIndex = left;
}
if (right < len && arr[right] > arr[maxIndex]) {
maxIndex = right;
}
// 如果最大值不是父节点
if (maxIndex != i) {
// 则交换父节点和最大值的的位置
swap(arr, maxIndex, i);
// 递归调用adjustHeap()进行调整
adjustHeap(arr, maxIndex, len);
}
}
// 交换位置
private static void swap(int[] arr, int a, int b) {
int temp = arr[a];
arr[a] = arr[b];
arr[b] = temp;
}
}
```
优化后的代码在算法效率和代码可读性上都有所提升,建议在实际开发中采用这种方式进行堆构建。
相关推荐
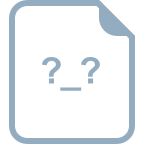
















