python pymysql中增加状态检测与报警处理语句
时间: 2024-05-24 18:11:08 浏览: 69
以下是一个基于Python pymysql库的增加状态检测与报警处理的示例代码:
import pymysql
import time
import smtplib
from email.mime.text import MIMEText
from email.header import Header
# 数据库连接配置
db_config = {
'host': 'localhost',
'user': 'root',
'password': '123456',
'database': 'testdb',
'charset': 'utf8mb4',
'cursorclass': pymysql.cursors.DictCursor
}
# 邮箱配置
mail_config = {
'server': 'smtp.qq.com',
'port': 465,
'username': 'your_email@qq.com',
'password': 'your_email_password',
'sender': 'your_email@qq.com',
'receiver': 'receiver_email@qq.com'
}
# 定义检测函数
def check_status():
try:
# 连接数据库
connection = pymysql.connect(**db_config)
# 获取游标
with connection.cursor() as cursor:
# 执行查询语句
sql = 'SELECT COUNT(*) FROM `users`'
cursor.execute(sql)
result = cursor.fetchone()
# 判断结果是否为None
if result is None:
# 发送报警邮件
send_mail('数据库查询出错,请尽快处理!')
except Exception as e:
# 发送报警邮件
send_mail('数据库连接出错,请尽快处理!')
finally:
# 关闭连接
connection.close()
# 定义发送邮件函数
def send_mail(content):
try:
# 邮件内容
message = MIMEText(content, 'plain', 'utf-8')
message['From'] = Header('Python程序', 'utf-8')
message['To'] = Header('管理员', 'utf-8')
message['Subject'] = Header('程序报警', 'utf-8')
# 连接SMTP服务器
smtp_server = smtplib.SMTP_SSL(mail_config['server'], mail_config['port'])
smtp_server.login(mail_config['username'], mail_config['password'])
# 发送邮件
smtp_server.sendmail(mail_config['sender'], mail_config['receiver'], message.as_string())
except Exception as e:
print('邮件发送失败:', e)
finally:
# 关闭连接
smtp_server.quit()
# 定义主函数
def main():
while True:
# 每隔一分钟检测一次状态
check_status()
time.sleep(60)
if __name__ == '__main__':
main()
该代码中,check_status()函数用于检测数据库状态,如果出现异常情况就发送报警邮件;send_mail()函数用于发送邮件;main()函数用于循环调用check_status()函数,并在每次调用之间休眠60秒。在该示例中,我们只是检测了数据库查询语句的执行情况,实际应用中可以根据需要检测更多状态,并进行相应处理。
阅读全文
相关推荐
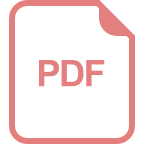
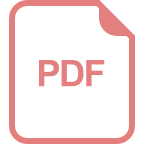
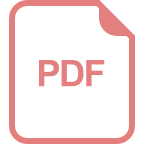
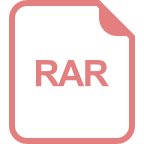
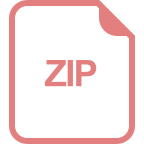
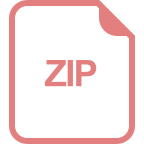
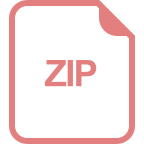
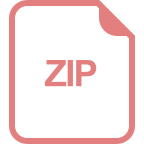
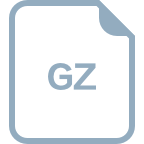
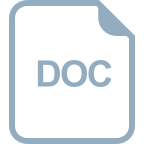
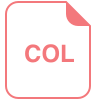
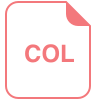
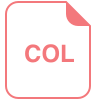
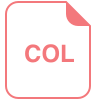
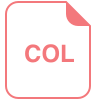
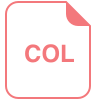
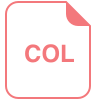
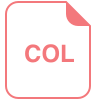
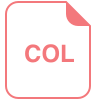