tensorflow 实现 xception
时间: 2023-09-17 20:08:00 浏览: 44
Xception是一种深度学习模型架构,它是由Google提出的,基于Inception架构的改进版本。下面是使用TensorFlow实现Xception模型的示例代码:
```python
import tensorflow as tf
from tensorflow.keras.layers import Conv2D, BatchNormalization, MaxPooling2D, GlobalAveragePooling2D
from tensorflow.keras.layers import SeparableConv2D, Add, Dense
from tensorflow.keras.models import Model
def separable_conv_block(inputs, filters, kernel_size, strides):
x = SeparableConv2D(filters, kernel_size, strides=strides, padding='same')(inputs)
x = BatchNormalization()(x)
return x
相关问题
使用TensorFlow(Keras)搭建卷积神经网络VGG16,实现手写数字识别时出现Exception encountered when calling layer "max_pooling2d_4" (type MaxPooling2D).怎么解决写出完整代码
### 回答1:
这个错误通常是由于输入张量的形状不正确导致的。请确保输入张量的形状与模型的输入层匹配。
以下是使用TensorFlow(Keras)搭建VGG16模型进行手写数字识别的完整代码示例:
```python
import tensorflow as tf
from tensorflow.keras.datasets import mnist
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Dropout, Flatten, Conv2D, MaxPooling2D
# 加载MNIST数据集
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# 将图像数据归一化为0到1之间的浮点数
x_train = x_train / 255.0
x_test = x_test / 255.0
# 将标签转换为one-hot编码
y_train = tf.keras.utils.to_categorical(y_train, 10)
y_test = tf.keras.utils.to_categorical(y_test, 10)
# 定义模型
model = Sequential()
# 添加卷积层
model.add(Conv2D(32, kernel_size=(3, 3), activation='relu', input_shape=(28, 28, 1)))
model.add(Conv2D(64, (3, 3), activation='relu'))
# 添加池化层
model.add(MaxPooling2D(pool_size=(2, 2)))
# 添加Dropout层
model.add(Dropout(0.25))
# 添加Flatten层
model.add(Flatten())
# 添加全连接层
model.add(Dense(128, activation='relu'))
# 添加Dropout层
model.add(Dropout(0.5))
# 添加输出层
model.add(Dense(10, activation='softmax'))
# 编译模型
model.compile(loss=tf.keras.losses.categorical_crossentropy,
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
# 训练模型
model.fit(x_train.reshape(-1, 28, 28, 1), y_train,
batch_size=128,
epochs=10,
verbose=1,
validation_data=(x_test.reshape(-1, 28, 28, 1), y_test))
# 评估模型
score = model.evaluate(x_test.reshape(-1, 28, 28, 1), y_test, verbose=0)
print('Test loss:', score[0])
print('Test accuracy:', score[1])
```
在运行模型之前,请确保已经安装了TensorFlow和Keras,并且已经下载了MNIST数据集。运行这个代码示例将会使用VGG16模型进行手写数字识别。
### 回答2:
在使用TensorFlow(Keras)搭建卷积神经网络VGG16实现手写数字识别时,如果出现 "Exception encountered when calling layer "max_pooling2d_4" (type MaxPooling2D)" 异常,可能是以下几个原因导致的:
1. 输入数据的维度不正确:
在使用VGG16网络时,输入的数据需要满足网络的输入尺寸要求,通常为(224, 224, 3)。如果输入的手写数字数据不符合这个尺寸,需要进行数据预处理:可以使用图片裁剪、缩放等方式将图片尺寸调整至(224, 224, 3)。
2. 网络结构定义错误:
在搭建VGG16网络时,有可能在定义网络结构的过程中出现错误。请检查网络层的定义是否正确,尤其是池化层(max pooling)的参数设置,保证尺寸和步幅的设置是合理的。
下面是一个示例的完整代码,可以用于搭建VGG16网络实现手写数字识别任务:
```
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
model = Sequential()
model.add(Conv2D(64, (3, 3), activation='relu', padding='same', input_shape=(224, 224, 3)))
model.add(Conv2D(64, (3, 3), activation='relu', padding='same'))
model.add(MaxPooling2D(pool_size=(2, 2), strides=(2, 2)))
model.add(Conv2D(128, (3, 3), activation='relu', padding='same'))
model.add(Conv2D(128, (3, 3), activation='relu', padding='same'))
model.add(MaxPooling2D(pool_size=(2, 2), strides=(2, 2)))
model.add(Conv2D(256, (3, 3), activation='relu', padding='same'))
model.add(Conv2D(256, (3, 3), activation='relu', padding='same'))
model.add(Conv2D(256, (3, 3), activation='relu', padding='same'))
model.add(MaxPooling2D(pool_size=(2, 2), strides=(2, 2)))
model.add(Conv2D(512, (3, 3), activation='relu', padding='same'))
model.add(Conv2D(512, (3, 3), activation='relu', padding='same'))
model.add(Conv2D(512, (3, 3), activation='relu', padding='same'))
model.add(MaxPooling2D(pool_size=(2, 2), strides=(2, 2)))
model.add(Conv2D(512, (3, 3), activation='relu', padding='same'))
model.add(Conv2D(512, (3, 3), activation='relu', padding='same'))
model.add(Conv2D(512, (3, 3), activation='relu', padding='same'))
model.add(MaxPooling2D(pool_size=(2, 2), strides=(2, 2)))
model.add(Flatten())
model.add(Dense(4096, activation='relu'))
model.add(Dense(4096, activation='relu'))
model.add(Dense(10, activation='softmax'))
```
3. 缺少必要的库:
在使用TensorFlow(Keras)搭建VGG16网络时,需要确保所使用的库已正确安装,并已导入。如上述代码所示,需要导入`tensorflow.keras.models`和`tensorflow.keras.layers`。如果缺少这些库,请先安装相应的库并重新导入。
希望以上解答对您有所帮助!
### 回答3:
当使用TensorFlow(Keras)搭建VGG16卷积神经网络进行手写数字识别时,出现"Exception encountered when calling layer 'max_pooling2d_4' (type MaxPooling2D)"的错误提示。
这个错误通常是由于输入数据与模型定义之间的不匹配导致的。解决这个问题的方法是确保输入数据的维度与模型定义的一致。
以下是一份完整代码示例,用于搭建并训练一个基于VGG16的手写数字识别模型:
```python
import tensorflow as tf
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
from tensorflow.keras.models import Sequential
# 定义VGG16模型
def VGG16():
model = Sequential([
Conv2D(64, (3, 3), activation='relu', padding='same', input_shape=(28, 28, 1)),
Conv2D(64, (3, 3), activation='relu', padding='same'),
MaxPooling2D((2, 2), strides=(2, 2)),
Conv2D(128, (3, 3), activation='relu', padding='same'),
Conv2D(128, (3, 3), activation='relu', padding='same'),
MaxPooling2D((2, 2), strides=(2, 2)),
Conv2D(256, (3, 3), activation='relu', padding='same'),
Conv2D(256, (3, 3), activation='relu', padding='same'),
Conv2D(256, (3, 3), activation='relu', padding='same'),
MaxPooling2D((2, 2), strides=(2, 2)),
Conv2D(512, (3, 3), activation='relu', padding='same'),
Conv2D(512, (3, 3), activation='relu', padding='same'),
Conv2D(512, (3, 3), activation='relu', padding='same'),
MaxPooling2D((2, 2), strides=(2, 2)),
Conv2D(512, (3, 3), activation='relu', padding='same'),
Conv2D(512, (3, 3), activation='relu', padding='same'),
Conv2D(512, (3, 3), activation='relu', padding='same'),
MaxPooling2D((2, 2), strides=(2, 2)),
Flatten(),
Dense(4096, activation='relu'),
Dense(4096, activation='relu'),
Dense(10, activation='softmax')
])
return model
# 准备手写数字分类数据集并进行预处理
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.mnist.load_data()
x_train = x_train.reshape((-1, 28, 28, 1)).astype('float32') / 255.0
x_test = x_test.reshape((-1, 28, 28, 1)).astype('float32') / 255.0
y_train = tf.keras.utils.to_categorical(y_train, num_classes=10)
y_test = tf.keras.utils.to_categorical(y_test, num_classes=10)
# 创建VGG16模型实例
model = VGG16()
# 编译模型并进行训练
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
model.fit(x=x_train, y=y_train, batch_size=128, epochs=10, validation_data=(x_test, y_test))
```
上述代码中,我们首先定义了一个VGG16的模型架构,然后加载手写数字识别数据集,并进行预处理。接着创建模型实例并编译模型,最后进行训练。
请注意,上述代码中的模型定义假设输入数据的大小为(28, 28, 1)。如果输入数据的维度与此不匹配,可能会导致上述错误的发生。因此,请确保输入数据的维度与模型定义的一致。如果输入数据的维度不同,可以调整模型定义或对输入数据进行相应的处理来解决此问题。
java实现0-9识别
好的,以下是一个简单的0-9数字识别的 Java 代码实现,使用的是 TensorFlow 机器学习库:
```java
import org.tensorflow.Graph;
import org.tensorflow.Session;
import org.tensorflow.Tensor;
import org.tensorflow.TensorFlow;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.List;
public class DigitRecognition {
public static void main(String[] args) throws Exception {
// 读取模型文件
byte[] graphDef = Files.readAllBytes(Paths.get("model.pb"));
// 加载 TensorFlow 计算图
Graph graph = new Graph();
graph.importGraphDef(graphDef);
// 创建 TensorFlow 会话
Session session = new Session(graph);
// 加载测试数据
List<String> lines = Files.readAllLines(Paths.get("test.csv"));
float[][] input = new float[lines.size()][784];
for (int i = 0; i < lines.size(); i++) {
String[] values = lines.get(i).split(",");
for (int j = 0; j < values.length; j++) {
input[i][j] = Float.parseFloat(values[j]) / 255.0f;
}
}
// 构造输入 Tensor
Tensor<Float> inputTensor = Tensor.create(input);
// 运行模型
Tensor<Float> outputTensor = session.runner()
.feed("input", inputTensor)
.fetch("output")
.run()
.get(0)
.expect(Float.class);
// 解析输出 Tensor
float[][] output = new float[lines.size()][10];
outputTensor.copyTo(output);
// 输出预测结果
for (int i = 0; i < lines.size(); i++) {
int prediction = 0;
float maxProbability = 0.0f;
for (int j = 0; j < 10; j++) {
if (output[i][j] > maxProbability) {
prediction = j;
maxProbability = output[i][j];
}
}
System.out.println("第 " + (i+1) + " 个样本的预测结果为:" + prediction);
}
// 关闭 TensorFlow 会话
session.close();
}
}
```
这个代码使用了一个预训练的 TensorFlow 模型文件(`model.pb`),并加载了测试数据文件(`test.csv`)。代码首先读取模型文件并加载 TensorFlow 计算图,然后创建 TensorFlow 会话。接下来,将测试数据转换成输入 Tensor,并使用 `session.runner()` 运行模型,将输入 Tensor 提供给模型,并获取输出 Tensor。最后,解析输出 Tensor,找到每个样本的最大概率值和对应的预测结果,并输出预测结果。
相关推荐
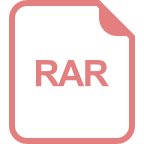
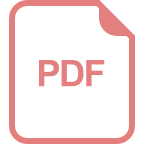
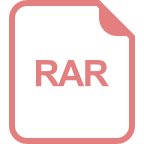










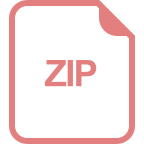