解释代码public class Book { private String name; public Book(String name) { this.name = name; } public String getName() { return name; } }
时间: 2023-05-17 12:04:20 浏览: 148
这段代码定义了一个名为 Book 的类,其中包含一个私有的字符串类型的属性 name 和一个公共的构造函数 Book(String name),用于初始化 name 属性。同时,还有一个公共的方法 getName(),用于获取 name 属性的值。
相关问题
package com.demo.bean; public class Book {//教材 private long id; private String bookId;//教材ID private String name;//教材名称 private String price;//价格 private String author;//编者 private String publisher;//出版社 private String profession;//专业 private String channel;//渠道 private String appendix;//备注 public Book() {} public Book(long id, String bookId, String name, String price, String author, String publisher, String profession, String channel, String appendix) { this.id = id; this.bookId = bookId; this.name = name; this.price = price; this.author = author; this.publisher = publisher; this.profession = profession; this.channel = channel; this.appendix = appendix; } public long getId() { return id; } public void setId(long id) { this.id = id; } public String getBookId() { return bookId; } public void setBookId(String bookId) { this.bookId = bookId; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getPrice() { return price; } public void setPrice(String price) { this.price = price; } public String getAuthor() { return author; } public void setAuthor(String author) { this.author = author; } public String getPublisher() { return publisher; } public void setPublisher(String publisher) { this.publisher = publisher; } public String getProfession() { return profession; } public void setProfession(String profession) { this.profession = profession; } public String getChannel() { return channel; } public void setChannel(String channel) { this.channel = channel; } public String getAppendix() { return appendix; } public void setAppendix(String appendix) { this.appendix = appendix; } @Override public String toString() { return "Book [id=" + id + ", bookId=" + bookId + ", name=" + name + ", price=" + price + ", author=" + author + ",
publisher=" + publisher + ", profession=" + profession + ", channel=" + channel + ", appendix=" + appendix + "]"; } }
这是一个 Java 类,表示一个教材(Book)对象,包括教材的 ID、名称、价格、编者、出版社、专业、渠道和备注等属性。其中包含了一个无参构造函数和一个有参构造函数,以及对应的 getter 和 setter 方法。此外,还重写了 toString 方法,以便于在需要打印该对象时,可以直接输出其属性值。
现有类Person和Book,其定义如下: import java.io.Serializable; import java.time.LocalDate; public class Person implements Serializable{ private static final long serialVersionUID = 1L; private String name ; private String gender ; private LocalDate birthday ; private String biography ; public Person() { } public Person(String name , String gender , String biography , int year , int month ,int day) { this.name = name ; this.gender = gender ; this.biography = biography ; this.birthday = LocalDate.of(year , month , day) ; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getGender() { return gender; } public void setGender(String gender) { this.gender = gender; } public LocalDate getBirthday() { return birthday; } public void setBirthday(LocalDate birthday) { this.birthday = birthday; } public String getBiography() { return biography; } public void setBiography(String biography) { this.biography = biography; } @Override public String toString() { return "name: " + name + " , gender: " + gender + " , birthday: " + birthday + " , biography: " + biography ; } } import java.io.Serializable; public class Book implements Serializable{ private static final long serialVersionUID = 1L; private String name; private Person author; private int price; public String getName() { return name; } public void setName(String name) { this.name = name; } public Person getAuthor() { return author; } public void setAuthor(Person author) { this.author = author; } public int getPrice() { return price; } public void setPrice(int price) { this.price = price; } public Book() { } public Book(String name,Person author,int price) { this.name = name; this.author = author; this.price = price; } @Override public String toString() { return "name: " + name + "\nauthor: " + author + "\nprice: " + price ; } } 有一段程序用objectOutputStream的writeobject()方法连续向文件dict.dic中写入了5个Book类型的对象。现请你写一段程序将这5个对象读出来。 注意:你的程序中要把Person和Book类的定义复制过去。
可以使用ObjectInputStream的readObject()方法从文件中读取序列化后的对象。具体代码如下:
```java
import java.io.FileInputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.util.ArrayList;
import java.util.List;
public class ReadBooks {
public static void main(String[] args) {
String filename = "dict.dic";
List<Book> books = new ArrayList<>();
try (ObjectInputStream ois = new ObjectInputStream(new FileInputStream(filename))) {
for (int i = 0; i < 5; i++) {
Book book = (Book) ois.readObject();
books.add(book);
}
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
for (Book book : books) {
System.out.println(book);
}
}
}
```
在上面的代码中,首先定义了一个文件名filename和一个空的List<Book> books,然后使用ObjectInputStream从文件中读取5个Book对象并添加到List中。最后遍历List并打印每个Book对象的信息。
阅读全文
相关推荐
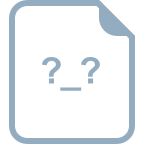
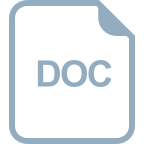














