python着色点代码
时间: 2023-02-08 09:53:26 浏览: 295
下面是一个简单的 Python 代码示例,使用 Matplotlib 绘制一个带有点的散点图并对点进行着色:
```
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
colors = ['red', 'green', 'blue', 'purple', 'yellow']
plt.scatter(x, y, c=colors)
plt.show()
```
上面的代码使用 Matplotlib 绘制了一个带有 5 个点的散点图。点的横坐标和纵坐标分别为 x 和 y 列表,而点的颜色则由 colors 列表提供。'c'是 color 的缩写 可以用来表示颜色
使用scatter 这个函数可以绘制散点图,可以用来绘制点图
请注意,在运行此代码之前,需要确保已经安装了 Matplotlib 库。
相关问题
生成python地图着色问题代码
Sure, I can help you with that. Here's an example code for generating a colored map in Python using the Matplotlib library:
```python
import numpy as np
import matplotlib.pyplot as plt
# Define the data
values = np.random.rand(10, 10)
# Define the colormap
cmap = plt.get_cmap('Greens')
# Plot the data with the colormap
plt.imshow(values, cmap=cmap)
# Show the plot
plt.show()
```
This code creates a random 10x10 array of values and uses the 'Greens' colormap to plot it as a colored map. You can modify the size of the array and use a different colormap to fit your specific needs. Let me know if you need further assistance.
获取每一个点云的RGB值并重新着色python代码
要获取每个点云的RGB值并重新着色,你需要首先获取点云数据,然后针对每个点的RGB值进行处理。以下是一个示例代码:
```python
import numpy as np
def recolor_point_cloud(point_cloud):
# 获取RGB值
colors = point_cloud[:, 3:]
# 计算每个点的颜色大小,这里使用RGB值的总和
color_sizes = np.sum(colors, axis=1)
# 对颜色大小进行排序,返回排序后的索引
sorted_indices = np.argsort(color_sizes)
# 根据排名分配新的颜色值
num_points = len(point_cloud)
new_colors = np.zeros_like(colors)
for i, index in enumerate(sorted_indices):
# 这里可以自定义新的颜色值,例如根据排序位置生成渐变色
new_colors[i] = [index/num_points, 1 - index/num_points, 0]
# 更新点云的颜色值
point_cloud[:, 3:] = new_colors
return point_cloud
# 示例用法
# 假设point_cloud是一个N x 6的NumPy数组,其中前三列是点的坐标(x, y, z),后三列是RGB值(r, g, b)
# 调用recolor_point_cloud函数重新着色点云
recolored_point_cloud = recolor_point_cloud(point_cloud)
```
请注意,在上述示例中,我们使用排序后的索引来生成新的颜色值。你可以根据具体需求自定义生成新颜色的逻辑。另外,这个示例代码仅适用于每个点的RGB值存在于0到1之间的情况。如果RGB值范围不同,请根据实际情况进行归一化或者调整代码。
阅读全文
相关推荐
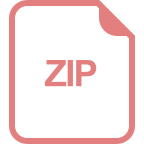
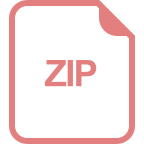


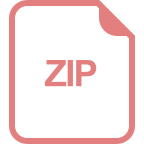
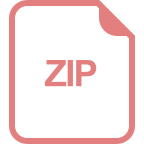
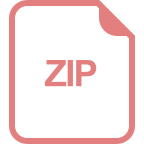
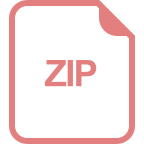
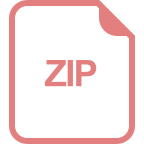
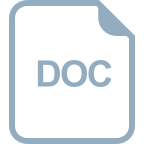


