QApplication 关闭窗口 进程还在运行
时间: 2023-07-14 08:13:12 浏览: 54
当你关闭一个Qt窗口时,QApplication并不会自动退出。这意味着你需要手动调用QApplication的quit()函数来终止应用程序。例如,在你的窗口的closeEvent()事件处理程序中添加以下代码:
```python
def closeEvent(self, event):
reply = QMessageBox.question(self, 'Message', "Are you sure you want to quit?", QMessageBox.Yes | QMessageBox.No, QMessageBox.No)
if reply == QMessageBox.Yes:
event.accept()
QApplication.quit()
else:
event.ignore()
```
当用户点击关闭按钮时,会显示一个消息框,询问用户是否真的要退出。如果用户确认退出,则调用QApplication的quit()函数来终止应用程序。
相关问题
python实现:实时将cmd窗口的每条数据显示在QPlainTextEdit窗口
以下是用Python实现实时将CMD窗口的每条数据显示在QPlainTextEdit窗口的示例代码:
```python
import sys
import subprocess
from PyQt5.QtWidgets import QApplication, QWidget, QVBoxLayout, QPlainTextEdit
class CmdWindow(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setWindowTitle('CMD Window')
self.setGeometry(100, 100, 640, 480)
# 创建QPlainTextEdit控件
self.text_edit = QPlainTextEdit(self)
self.text_edit.setReadOnly(True)
# 创建垂直布局
vbox = QVBoxLayout()
vbox.addWidget(self.text_edit)
# 设置窗口布局
self.setLayout(vbox)
# 启动CMD进程
self.cmd_process = subprocess.Popen('cmd.exe', stdout=subprocess.PIPE)
# 定时读取CMD输出
self.timer = self.startTimer(100)
def timerEvent(self, event):
# 读取CMD输出
output = self.cmd_process.stdout.readline()
# 判断输出是否为空
if output:
# 将输出转换为字符串类型
output_str = output.decode('gbk').strip()
# 将输出添加到QPlainTextEdit控件中
self.text_edit.appendPlainText(output_str)
else:
# 如果输出为空,则停止定时器
self.killTimer(self.timer)
if __name__ == '__main__':
app = QApplication(sys.argv)
window = CmdWindow()
window.show()
sys.exit(app.exec_())
```
在上述示例代码中,我们首先创建了一个`CmdWindow`类,该类继承自`QWidget`类,表示一个窗口。
在`initUI`方法中,我们创建了一个`QPlainTextEdit`控件,并将其添加到窗口中。同时,我们启动了一个CMD进程,并使用`subprocess`模块的`Popen`方法来获取CMD进程的标准输出。
在`timerEvent`方法中,我们使用定时器来读取CMD进程的标准输出,并将输出添加到`QPlainTextEdit`控件中。在定时器的回调函数中,我们首先使用`stdout.readline()`方法读取CMD输出,然后判断输出是否为空。如果输出不为空,则将其转换为字符串类型,并添加到`QPlainTextEdit`控件中;如果输出为空,则停止定时器。
最后,我们创建了一个`QApplication`对象,并将`CmdWindow`窗口显示出来。当用户关闭窗口时,`QApplication`对象将退出,整个程序运行结束。
如何将python接受到的视频图像在C语言的Qt窗口中显示
要将Python接受到的视频图像在C语言的Qt窗口中显示,你可以使用以下步骤:
1. 在Python中使用OpenCV等库捕获视频帧。
2. 将视频帧转换为Qt支持的图像格式,如QImage。
3. 将QImage传递给C++的Qt程序,可以使用Socket或共享内存等方式进行进程间通信。
4. 在C++中使用Qt的界面库,在窗口中显示QImage。
具体实现方法可以参考以下步骤:
1. 在Python中使用OpenCV等库捕获视频帧:
```python
import cv2
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
# 处理帧
# 转换为Qt支持的图像格式
qimage = QImage(frame.data, frame.shape[1], frame.shape[0], QImage.Format_RGB888)
```
2. 将QImage传递给C++的Qt程序:
在Python中可以使用socket或共享内存等方式将QImage传递给C++的Qt程序。以下是使用socket方式实现的示例代码:
```python
import socket
import struct
def send_qimage(qimage):
# 创建socket
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# 连接服务器
sock.connect(('localhost', 8888))
# 转换为bytes格式
data = qimage.bits().asstring(qimage.byteCount())
# 打包数据
header = struct.pack('lll', qimage.width(), qimage.height(), len(data))
# 发送数据头
sock.send(header)
# 发送数据
sock.sendall(data)
# 关闭socket
sock.close()
```
在C++中可以使用socket编写服务器端程序,接收Python发送的QImage数据。
3. 在C++中使用Qt的界面库,在窗口中显示QImage:
在C++中可以使用Qt的界面库,在窗口中显示QImage。以下是显示QImage的示例代码:
```c++
#include <QtWidgets/QApplication>
#include <QtGui/QImage>
#include <QtGui/QPainter>
#include <QtGui/QPixmap>
#include <QtGui/QWindow>
#include <QtNetwork/QTcpServer>
#include <QtNetwork/QTcpSocket>
#include <iostream>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
// 创建窗口
QWidget window;
window.show();
// 创建服务器
QTcpServer server;
server.listen(QHostAddress::Any, 8888);
// 监听连接请求
QObject::connect(&server, &QTcpServer::newConnection, [&](){
// 接受连接
QTcpSocket *socket = server.nextPendingConnection();
QObject::connect(socket, &QTcpSocket::readyRead, [=](){
// 读取数据头
char header[12];
socket->read(header, sizeof(header));
int width, height, size;
std::memcpy(&width, header, 4);
std::memcpy(&height, header + 4, 4);
std::memcpy(&size, header + 8, 4);
// 读取数据
QByteArray data = socket->read(size);
QImage qimage((const uchar *)data.constData(), width, height, QImage::Format_RGB888);
// 在窗口中显示
QPixmap pixmap = QPixmap::fromImage(qimage);
QPainter painter(&window);
painter.drawPixmap(0, 0, pixmap);
});
});
// 运行应用程序
return app.exec();
}
```
以上代码中,服务器监听端口8888,并在接收到Python发送的QImage数据时,在窗口中显示QImage。
相关推荐
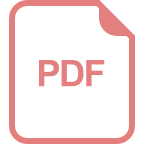








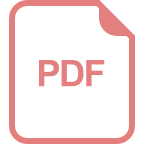
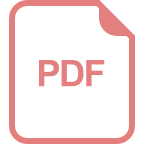
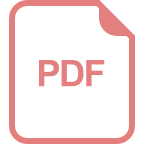
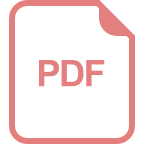
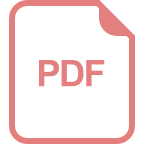