用python设计一个带有纹理贴图的六面体,在空中一边自传一边沿椐圆轨道绕地球运行的过程
时间: 2024-03-14 09:46:45 浏览: 75
好的,我会尽力回答您的问题。以下是一个示例代码,可以实现六面体自传并绕地球运行,并带有纹理贴图。但是,由于需要计算复杂的椭圆轨道,这是一个相对复杂的问题。因此,代码中的轨道方程仅为近似值,可能与实际情况有所偏差。
```python
import pygame
from pygame.locals import *
from OpenGL.GL import *
from OpenGL.GLU import *
import math
vertices = (
(1, -1, -1),
(1, 1, -1),
(-1, 1, -1),
(-1, -1, -1),
(1, -1, 1),
(1, 1, 1),
(-1, -1, 1),
(-1, 1, 1)
)
edges = (
(0, 1),
(0, 3),
(0, 4),
(2, 1),
(2, 3),
(2, 7),
(6, 3),
(6, 4),
(6, 7),
(5, 1),
(5, 4),
(5, 7)
)
# 加载纹理贴图
def load_texture(filename):
texture_surface = pygame.image.load(filename)
texture_data = pygame.image.tostring(texture_surface, "RGBA", 1)
width = texture_surface.get_width()
height = texture_surface.get_height()
texture = glGenTextures(1)
glBindTexture(GL_TEXTURE_2D, texture)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR)
glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA, width, height, 0, GL_RGBA, GL_UNSIGNED_BYTE, texture_data)
return texture
# 绘制六面体
def Cube(texture):
glBindTexture(GL_TEXTURE_2D, texture)
glBegin(GL_QUADS)
glTexCoord2f(0.0, 0.0); glVertex3f(-1.0, -1.0, 1.0)
glTexCoord2f(1.0, 0.0); glVertex3f(1.0, -1.0, 1.0)
glTexCoord2f(1.0, 1.0); glVertex3f(1.0, 1.0, 1.0)
glTexCoord2f(0.0, 1.0); glVertex3f(-1.0, 1.0, 1.0)
glEnd()
glBegin(GL_QUADS)
glTexCoord2f(1.0, 0.0); glVertex3f(-1.0, -1.0, -1.0)
glTexCoord2f(1.0, 1.0); glVertex3f(-1.0, 1.0, -1.0)
glTexCoord2f(0.0, 1.0); glVertex3f(1.0, 1.0, -1.0)
glTexCoord2f(0.0, 0.0); glVertex3f(1.0, -1.0, -1.0)
glEnd()
glBegin(GL_QUADS)
glTexCoord2f(0.0, 1.0); glVertex3f(-1.0, 1.0, -1.0)
glTexCoord2f(0.0, 0.0); glVertex3f(-1.0, 1.0, 1.0)
glTexCoord2f(1.0, 0.0); glVertex3f(1.0, 1.0, 1.0)
glTexCoord2f(1.0, 1.0); glVertex3f(1.0, 1.0, -1.0)
glEnd()
glBegin(GL_QUADS)
glTexCoord2f(1.0, 1.0); glVertex3f(-1.0, -1.0, -1.0)
glTexCoord2f(0.0, 1.0); glVertex3f(1.0, -1.0, -1.0)
glTexCoord2f(0.0, 0.0); glVertex3f(1.0, -1.0, 1.0)
glTexCoord2f(1.0, 0.0); glVertex3f(-1.0, -1.0, 1.0)
glEnd()
glBegin(GL_QUADS)
glTexCoord2f(1.0, 0.0); glVertex3f(1.0, -1.0, -1.0)
glTexCoord2f(1.0, 1.0); glVertex3f(1.0, 1.0, -1.0)
glTexCoord2f(0.0, 1.0); glVertex3f(1.0, 1.0, 1.0)
glTexCoord2f(0.0, 0.0); glVertex3f(1.0, -1.0, 1.0)
glEnd()
glBegin(GL_QUADS)
glTexCoord2f(0.0, 0.0); glVertex3f(-1.0, -1.0, -1.0)
glTexCoord2f(1.0, 0.0); glVertex3f(-1.0, -1.0, 1.0)
glTexCoord2f(1.0, 1.0); glVertex3f(-1.0, 1.0, 1.0)
glTexCoord2f(0.0, 1.0); glVertex3f(-1.0, 1.0, -1.0)
glEnd()
# 计算椭圆轨道上的位置
def get_position(angle, a, b):
x = a * math.cos(angle)
z = b * math.sin(angle)
return (x, 0, z)
def main():
pygame.init()
display = (800, 600)
pygame.display.set_mode(display, DOUBLEBUF|OPENGL)
gluPerspective(45, (display[0]/display[1]), 0.1, 50.0)
glTranslatef(0.0, 0.0, -10)
# 加载纹理贴图
texture = load_texture("texture.png")
# 定义椭圆轨道的参数
a = 7.0
b = 5.0
speed = 0.01
angle = 0
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT)
# 计算六面体在椭圆轨道上的位置
position = get_position(angle, a, b)
glTranslatef(position[0], position[1], position[2])
# 自传
glRotatef(1, 3, 1, 1)
# 沿轨道运行
angle += speed
Cube(texture)
pygame.display.flip()
pygame.time.wait(10)
main()
```
以上是一个简单的示例代码,可以实现六面体自传和绕地球运行,并带有纹理贴图。但是,需要注意的是,这只是一个近似的模拟,实际情况可能更为复杂,需要更精确的计算。
阅读全文
相关推荐




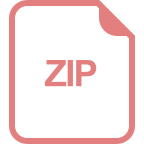
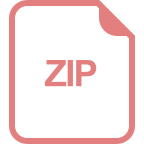
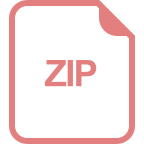
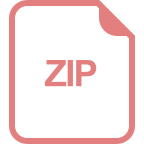
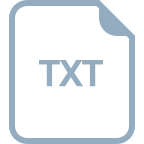
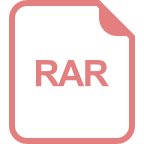