response = requests.post(url, json=payload) self.assertEqual(response.status_code, 201)
时间: 2024-05-20 15:18:44 浏览: 135
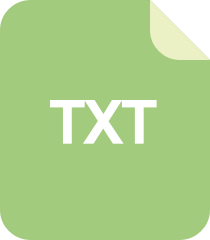
request 和response的用法
这段代码使用 Python 的 requests 库向指定的 URL 发送一个 POST 请求,并且发送了一个 JSON 格式的 payload。然后使用 assertEqual 方法断言该请求的返回状态码为 201。通常情况下,HTTP 201 状态码表示服务器成功处理了请求,并且创建了一个新的资源。
阅读全文
相关推荐
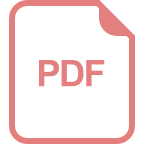
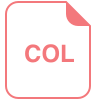
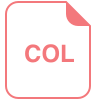
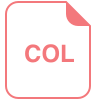
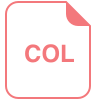
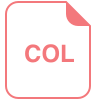
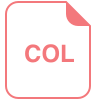
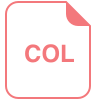
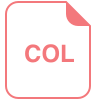
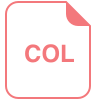

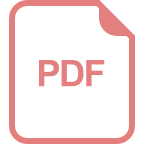
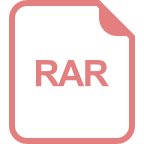
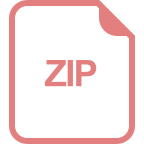