基于rt-thread操作系统的地址滑坡检测节点核心代码实现
时间: 2023-08-13 18:03:56 浏览: 51
以下是基于 RT-Thread 操作系统的地址滑坡检测节点核心代码实现的示例,仅供参考:
```c
#include <rtthread.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
/* 定义线程栈 */
#define THREAD_STACK_SIZE 1024
static rt_uint8_t thread_stack[THREAD_STACK_SIZE];
/* 定义消息队列 */
#define QUEUE_SIZE 20
static rt_mq_t gps_queue = RT_NULL;
static rt_mq_t acc_queue = RT_NULL;
static rt_mq_t upload_queue = RT_NULL;
/* 定义互斥锁 */
static rt_mutex_t mutex = RT_NULL;
/* 定义信号量 */
static rt_sem_t sem = RT_NULL;
/* 定义三轴传感器阈值 */
#define ACC_THRESHOLD 10
/* 定义 GPS 传感器数据结构 */
struct gps_data {
rt_uint8_t hour;
rt_uint8_t minute;
rt_uint8_t second;
rt_uint8_t latitude[10];
rt_uint8_t longitude[10];
};
/* 定义三轴传感器数据结构 */
struct acc_data {
rt_uint16_t x;
rt_uint16_t y;
rt_uint16_t z;
};
/* 采集 GPS 数据线程 */
static void collect_gps_data_thread(void* parameter)
{
struct gps_data gps;
while (1) {
/* 从 GPS 传感器中读取数据 */
/* ... */
/* 将数据发送到上传数据线程的消息队列中 */
if (rt_mq_send(upload_queue, &gps, sizeof(struct gps_data)) != RT_EOK) {
rt_kprintf("Failed to send GPS data to upload thread!\n");
}
/* 等待 1 秒 */
rt_thread_mdelay(1000);
}
}
/* 采集三轴传感器数据线程 */
static void collect_acc_data_thread(void* parameter)
{
struct acc_data acc;
while (1) {
/* 从 MPU6050 三轴传感器中读取数据 */
/* ... */
/* 将数据发送到阈值检测线程的消息队列中 */
if (rt_mq_send(acc_queue, &acc, sizeof(struct acc_data)) != RT_EOK) {
rt_kprintf("Failed to send accelerometer data to threshold thread!\n");
}
/* 等待 10 毫秒 */
rt_thread_mdelay(10);
}
}
/* 上传数据线程 */
static void upload_data_thread(void* parameter)
{
struct gps_data gps;
struct acc_data acc;
while (1) {
/* 从上传数据线程的消息队列中获取数据 */
if (rt_mq_recv(upload_queue, &gps, sizeof(struct gps_data), RT_WAITING_FOREVER) == sizeof(struct gps_data)) {
/* 将 GPS 数据上传到 Onenet 云平台 */
/* ... */
}
if (rt_mq_recv(upload_queue, &acc, sizeof(struct acc_data), RT_WAITING_FOREVER) == sizeof(struct acc_data)) {
/* 将三轴传感器数据上传到 Onenet 云平台 */
/* ... */
}
}
}
/* 按键处理线程 */
static void key_process_thread(void* parameter)
{
while (1) {
/* 处理来自按键键盘的输入 */
/* ... */
/* 根据不同的按键设置相应的参数 */
/* ... */
/* 等待 1 秒 */
rt_thread_mdelay(1000);
}
}
/* 阈值检测线程 */
static void threshold_thread(void* parameter)
{
struct acc_data acc;
while (1) {
/* 从阈值检测线程的消息队列中获取三轴传感器数据 */
if (rt_mq_recv(acc_queue, &acc, sizeof(struct acc_data), RT_WAITING_FOREVER) == sizeof(struct acc_data)) {
/* 检测数据是否超过设定阈值 */
if (acc.x > ACC_THRESHOLD || acc.y > ACC_THRESHOLD || acc.z > ACC_THRESHOLD) {
/* 将数据发送到上传数据线程的消息队列中 */
if (rt_mq_send(upload_queue, &acc, sizeof(struct acc_data)) != RT_EOK) {
rt_kprintf("Failed to send accelerometer data to upload thread!\n");
}
} else {
/* 将数据发送到采集三轴传感器数据线程的消息队列中 */
if (rt_mq_send(acc_queue, &acc, sizeof(struct acc_data)) != RT_EOK) {
rt_kprintf("Failed to send accelerometer data to collect thread!\n");
}
}
}
/* 等待 10 毫秒 */
rt_thread_mdelay(10);
}
}
int main(void)
{
/* 初始化硬件组件 */
/* ... */
/* 初始化消息队列 */
gps_queue = rt_mq_create("GPS", sizeof(struct gps_data), QUEUE_SIZE, RT_IPC_FLAG_FIFO);
acc_queue = rt_mq_create("ACC", sizeof(struct acc_data), QUEUE_SIZE, RT_IPC_FLAG_FIFO);
upload_queue = rt_mq_create("UPLOAD", sizeof(struct gps_data) + sizeof(struct acc_data), QUEUE_SIZE, RT_IPC_FLAG_FIFO);
if (gps_queue == RT_NULL || acc_queue == RT_NULL || upload_queue == RT_NULL) {
rt_kprintf("Failed to create message queue!\n");
return -1;
}
/* 初始化互斥锁 */
mutex = rt_mutex_create("Mutex", RT_IPC_FLAG_FIFO);
if (mutex == RT_NULL) {
rt_kprintf("Failed to create mutex!\n");
return -1;
}
/* 初始化信号量 */
sem = rt_sem_create("Sem", 0, RT_IPC_FLAG_FIFO);
if (sem == RT_NULL) {
rt_kprintf("Failed to create semaphore!\n");
return -1;
}
/* 创建采集 GPS 数据线程 */
rt_thread_t gps_thread = rt_thread_create("GPS", collect_gps_data_thread, RT_NULL, THREAD_STACK_SIZE, 5, 10);
if (gps_thread == RT_NULL) {
rt_kprintf("Failed to create GPS thread!\n");
return -1;
}
rt_thread_startup(gps_thread);
/* 创建采集三轴传感器数据线程 */
rt_thread_t acc_thread = rt_thread_create("ACC", collect_acc_data_thread, RT_NULL, THREAD_STACK_SIZE, 6, 10);
if (acc_thread == RT_NULL) {
rt_kprintf("Failed to create accelerometer thread!\n");
return -1;
}
rt_thread_startup(acc_thread);
/* 创建上传数据线程 */
rt_thread_t upload_thread = rt_thread_create("UPLOAD", upload_data_thread, RT_NULL, THREAD_STACK_SIZE, 7, 10);
if (upload_thread == RT_NULL) {
rt_kprintf("Failed to create upload thread!\n");
return -1;
}
rt_thread_startup(upload_thread);
/* 创建按键处理线程 */
rt_thread_t key_thread = rt_thread_create("KEY", key_process_thread, RT_NULL, THREAD_STACK_SIZE, 4, 10);
if (key_thread == RT_NULL) {
rt_kprintf("Failed to create key thread!\n");
return -1;
}
rt_thread_startup(key_thread);
/* 创建阈值检测线程 */
rt_thread_t threshold_thread = rt_thread_create("THRESHOLD", threshold_thread, RT_NULL, THREAD_STACK_SIZE, 8, 10);
if (threshold_thread == RT_NULL) {
rt_kprintf("Failed to create threshold thread!\n");
return -1;
}
rt_thread_startup(threshold_thread);
/* 启动 RT-Thread 调度器 */
rt_thread_mdelay(RT_TICK_PER_SECOND);
rt_system_scheduler_start();
return 0;
}
```
在以上代码中,我们使用了 RT-Thread 提供的多线程支持、消息队列、互斥锁和信号量等功能,以实现节点的数据采集、上传和处理等功能。其中,学习者需要根据具体硬件组件和 Onenet 云平台等情况进行相应的调整和修改。
相关推荐
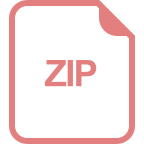
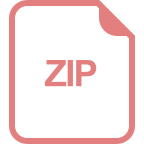







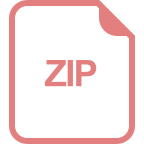
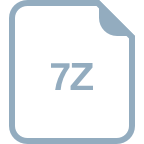
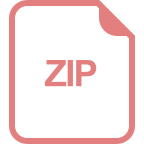
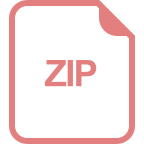
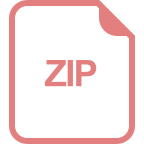
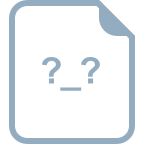
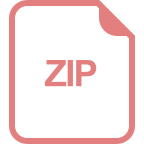