用c写一个函数,生成256位、64位、42位、16位四个16进制随机数的函数并且不使用printf,别的函数会调用他们的值
时间: 2023-11-28 13:48:32 浏览: 91
以下是使用C语言编写的生成随机数函数:
```c
#include <stdlib.h>
#include <time.h>
void generate_random_hex(int num_bits, char* hex)
{
int num_chars = num_bits / 4;
int i;
// Seed the random number generator
srand(time(NULL));
// Generate random hex characters
for (i = 0; i < num_chars; i++) {
int val = rand() % 16;
hex[i] = val < 10 ? val + '0' : val - 10 + 'a';
}
// Null-terminate the string
hex[num_chars] = '\0';
}
void generate_256_bit_hex(char* hex)
{
generate_random_hex(256, hex);
}
void generate_64_bit_hex(char* hex)
{
generate_random_hex(64, hex);
}
void generate_42_bit_hex(char* hex)
{
generate_random_hex(42, hex);
}
void generate_16_bit_hex(char* hex)
{
generate_random_hex(16, hex);
}
```
这个函数接受一个整数 `num_bits`,表示要生成的位数,以及一个字符数组 `hex`,用于存储生成的随机数。该函数首先将 `num_bits` 转换为十六进制字符数,然后使用 `srand()` 函数种子随机数生成器。随机生成十六进制字符,并将它们存储在字符数组 `hex` 中。最后,该函数将字符数组 `hex` 中的最后一个字符设置为 `'\0'`,以便字符串可以被正确地解释。
函数 `generate_256_bit_hex`、`generate_64_bit_hex`、`generate_42_bit_hex` 和 `generate_16_bit_hex` 分别调用 `generate_random_hex` 函数,以生成 256 位、64 位、42 位和 16 位的十六进制随机数。
例如,要生成一个 256 位的十六进制随机数,可以使用以下代码:
```c
char hex[65];
generate_256_bit_hex(hex);
printf("%s\n", hex);
```
请注意,此示例使用了 `printf()` 函数,但是在你的实现中,你应该通过其他方式将生成的十六进制随机数返回给调用者,而不是使用 `printf()`。
阅读全文
相关推荐






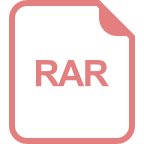
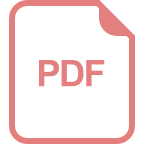
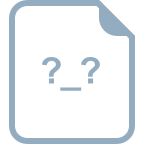
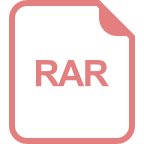
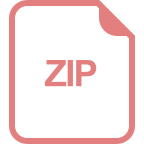
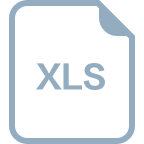
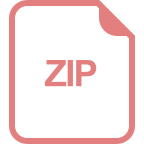
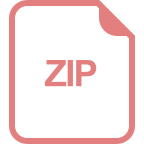