下列代码错误在哪:class person { public: person(int age) { m_age =new int(age); } //重载运算操作符 person& operator=(person &p) { if (m_age != NULL) { delete m_age; m_age = NULL; } //m_age = p.m_age;//浅拷贝 ////提供深拷贝解决浅拷贝问题: m_age = new int(*p.m_age); return *this; } ~person() { if (m_age != NULL) { delete m_age; m_age = NULL; } } int* m_age; }; void test01() { person p1(18); person p2(20); person p3(28); person p4 = p3; cout << "p1的年龄为:" << *p1.m_age << endl; cout << "p2的年龄为:" << *p2.m_age << endl; cout << "p3的年龄为:" << *p3.m_age << endl; cout << "p4的年龄为:" << *p4.m_age << endl; } int main() { test01(); }
时间: 2024-01-02 12:04:57 浏览: 24
这段代码存在潜在的内存泄漏问题。在 `operator=` 函数中,如果 `m_age` 不为 NULL,那么应该先释放它所指向的内存,再进行深拷贝。因为如果不释放 `m_age` 所指向的内存直接进行深拷贝,会导致原先指向的内存无法释放,从而造成内存泄漏。修改后的 `operator=` 函数如下:
```c++
person& operator=(person &p) {
if (this != &p) { // 避免自我赋值
if (m_age != NULL) {
delete m_age;
}
m_age = new int(*p.m_age);
}
return *this;
}
```
同时,在 `test01` 函数中,应该将 `p4` 的初始化方式改为使用 `p3` 赋值,即 `person p4 = p3;` 改为 `person p4(p3);`。因为前者会触发 `operator=` 函数,而后者会调用拷贝构造函数,这样更符合代码的语义。修改后的 `test01` 函数如下:
```c++
void test01() {
person p1(18);
person p2(20);
person p3(28);
person p4(p3);
cout << "p1的年龄为:" << *p1.m_age << endl;
cout << "p2的年龄为:" << *p2.m_age << endl;
cout << "p3的年龄为:" << *p3.m_age << endl;
cout << "p4的年龄为:" << *p4.m_age << endl;
}
```
相关推荐
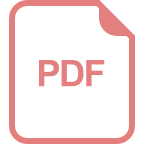
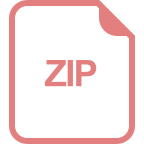
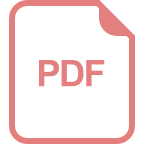















