学生数据要有学号、姓名、性别、专业、年级等,职工数据要有职工号、姓名、性别、年龄、部门、职称、工资等。要求将姓名、性别和数据输出设计成一个类person,学生类student和职工类employee,由类person来派生。要求程序完整(包含主函数)。
时间: 2023-12-28 08:17:47 浏览: 56
以下是完整的程序代码,包含主函数:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Person {
protected:
string name; // 姓名
char gender; // 性别
public:
Person(string n, char g) : name(n), gender(g) {}
virtual void display() {
cout << "姓名:" << name << endl;
cout << "性别:" << gender << endl;
}
};
class Student : public Person {
private:
string studentID; // 学号
string major; // 专业
string grade; // 年级
public:
Student(string n, char g, string id, string m, string gd)
: Person(n, g), studentID(id), major(m), grade(gd) {}
void display() {
Person::display();
cout << "学号:" << studentID << endl;
cout << "专业:" << major << endl;
cout << "年级:" << grade << endl;
}
};
class Employee : public Person {
private:
string employeeID; // 职工号
int age; // 年龄
string department; // 部门
string title; // 职称
double salary; // 工资
public:
Employee(string n, char g, string id, int a, string dept, string t, double sal)
: Person(n, g), employeeID(id), age(a), department(dept), title(t), salary(sal) {}
void display() {
Person::display();
cout << "职工号:" << employeeID << endl;
cout << "年龄:" << age << endl;
cout << "部门:" << department << endl;
cout << "职称:" << title << endl;
cout << "工资:" << salary << endl;
}
};
int main() {
Person *p1 = new Student("张三", 'M', "20210001", "计算机科学与技术", "大二");
Person *p2 = new Employee("李四", 'F', "1001", 35, "人力资源部", "经理", 10000.0);
p1->display();
cout << endl;
p2->display();
cout << endl;
delete p1;
delete p2;
return 0;
}
```
在上面的程序中,我们定义了一个基类`Person`,包含姓名和性别两个数据成员,还有一个`display()`虚函数用于输出信息。然后我们定义了两个派生类`Student`和`Employee`,分别表示学生和职工,它们都继承了`Person`类,同时还增加了一些额外的数据成员和`display()`函数用于输出信息。最后在主函数中,我们创建了一个`Person`类型的指针`p1`和`p2`,分别指向一个学生对象和一个职工对象,并调用它们的`display()`函数输出信息。注意,在最后必须释放内存,否则会产生内存泄漏。
阅读全文
相关推荐
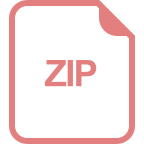
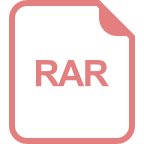
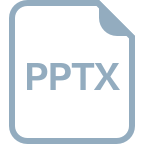















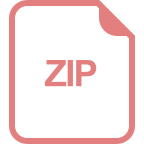