It is said that in ancient Indian holy temples, there was a game called Hanoi. The game is on a copper plate device with three rods (numbered a, B and C). there are many plates are placed in order from bottom to top and from large to small in rod A. The goal of the game: move all the gold plates on pole A to pole C, and keep the original order. Operating rules: only one plate can be moved at a time, and the large plate is always kept under the three rods and the small plate is on the top. During the operation, the plate can be placed on any rod A, B and C. Solve the above problems with programs, try to propose an optimized algorithm.
时间: 2024-02-29 19:51:29 浏览: 85
The Hanoi game is a classic problem in computer science and can be solved using recursion. Here is a Python implementation of the Hanoi game:
```python
def move(n, source, target, auxiliary):
if n > 0:
# Move n-1 plates from source to auxiliary using target as auxiliary
move(n-1, source, auxiliary, target)
# Move the largest plate from source to target
target.append(source.pop())
# Move the remaining n-1 plates from auxiliary to target using source as auxiliary
move(n-1, auxiliary, target, source)
# Example usage
source = [3, 2, 1]
target = []
auxiliary = []
move(len(source), source, target, auxiliary)
print(target)
```
The `move` function takes four arguments: `n` is the number of plates to move, `source` is the source rod, `target` is the target rod, and `auxiliary` is the auxiliary rod. The function recursively moves `n-1` plates from the source rod to the auxiliary rod using the target rod as an auxiliary, then moves the largest plate from the source rod to the target rod, and finally moves the `n-1` plates from the auxiliary rod to the target rod using the source rod as an auxiliary.
The optimized algorithm for the Hanoi game is to use an iterative approach instead of recursion. Here is a Python implementation of the iterative Hanoi game:
```python
def move(n, source, target, auxiliary):
if n % 2 == 0:
auxiliary, target = target, auxiliary
else:
source, target = target, source
for i in range(1, 2**n):
if i % 3 == 1:
source, target = target, source
elif i % 3 == 2:
source, auxiliary = auxiliary, source
else:
auxiliary, target = target, auxiliary
if i & (i-1) == 0:
plate = source.pop()
target.append(plate)
# Example usage
source = [3, 2, 1]
target = []
auxiliary = []
move(len(source), source, target, auxiliary)
print(target)
```
The `move` function takes the same arguments as before. The function first determines the order in which to move the plates based on the parity of `n` and the index of the plate. Then, the function uses a loop to move each plate in the correct order. Finally, the function checks if the current plate is the largest plate on the source rod and moves it to the target rod if it is. This approach is more efficient than the recursive approach because it avoids the overhead of function calls and stack frames.
阅读全文
相关推荐
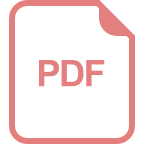
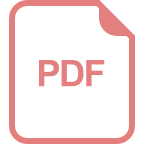
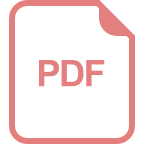
















