c++ boost 判断路径是否存在
时间: 2023-08-03 17:40:52 浏览: 145
在C++中,可以使用Boost库中的`filesystem`模块来判断路径是否存在。该模块提供了一组简单易用的API来处理文件和目录路径,包括检查文件或目录是否存在、创建目录、遍历目录等操作。
以下是一个示例代码,可以使用Boost库中的`exists()`函数判断指定的路径是否存在:
```c++
#include <boost/filesystem.hpp>
bool is_path_exist(const std::string& path)
{
boost::filesystem::path p(path);
return boost::filesystem::exists(p);
}
```
在上面的代码中,`boost::filesystem::path`用于存储路径信息,`boost::filesystem::exists()`函数用于检查指定的路径是否存在。该函数返回一个`bool`类型的值,如果指定路径存在则返回`true`,否则返回`false`。
需要注意的是,使用Boost库需要先安装Boost库并在编译时链接相应的库文件。
相关问题
c++ boost 判断路径是否存在 不存在就创建目录
可以使用Boost库中的`create_directory()`函数来创建目录。如果目录已经存在,则该函数不会做任何操作。
以下是一个示例代码,可以使用Boost库中的`exists()`函数和`create_directory()`函数判断指定的路径是否存在,如果不存在则创建目录:
```c++
#include <boost/filesystem.hpp>
bool is_path_exist(const std::string& path)
{
boost::filesystem::path p(path);
return boost::filesystem::exists(p);
}
bool create_directory_if_not_exist(const std::string& path)
{
if (!is_path_exist(path))
{
return boost::filesystem::create_directory(path);
}
else
{
return true;
}
}
```
在上面的代码中,`create_directory_if_not_exist()`函数会首先调用`is_path_exist()`函数来检查指定的路径是否存在。如果路径不存在,则调用`create_directory()`函数来创建目录。如果创建成功,则返回`true`,否则返回`false`。
需要注意的是,使用Boost库需要先安装Boost库并在编译时链接相应的库文件。
C++判断路径是否存在
在C++中,判断文件或目录路径是否存在通常涉及到文件系统操作。你可以使用标准库中的`std::filesystem`模块(自C++17开始引入),或者在旧版本中使用Boost.Filesystem库。以下是两种常见方法:
1. 使用C++17及以上版本的`std::filesystem`:
```cpp
#include <filesystem>
bool pathExists(const std::string& filePath) {
try {
std::filesystem::path p(filePath);
return std::filesystem::exists(p);
} catch (const std::filesystem::filesystem_error&) {
return false;
}
}
```
2. 使用Boost.Filesystem(适用于较早版本的C++):
```cpp
#include <boost/filesystem.hpp>
bool pathExists(const std::string& filePath) {
boost::filesystem::path p(filePath);
return boost::filesystem::exists(p);
}
```
这两种方法都尝试访问指定的路径,并检查它是否实际存在。如果路径不存在,会抛出异常或返回`false`。
阅读全文
相关推荐





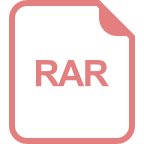

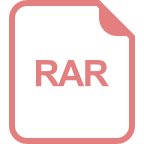








