在链串上实现判断两个串是否相等的功能
时间: 2023-12-20 13:07:43 浏览: 31
链串可以通过遍历每个节点,逐个比较每个字符来判断两个串是否相等。
具体实现步骤如下:
1. 判断两个链串的长度是否相等,如果不相等,则两个串一定不相等,直接返回 false。
2. 分别定义两个指针 p 和 q,分别指向两个链串的头节点。
3. 逐个比较每个节点的值是否相等,如果存在不相等的节点,则两个串不相等,直接返回 false。
4. 如果遍历完链串后,没有返回 false,则说明两个链串相等,返回 true。
以下是示例代码:
```c++
bool isEqual(LinkStrNode* s, LinkStrNode* t) {
int len1 = s->length;
int len2 = t->length;
if (len1 != len2) {
return false;
}
LinkStrNode *p = s->next;
LinkStrNode *q = t->next;
while (p != nullptr && q != nullptr) {
if (p->data != q->data) {
return false;
}
p = p->next;
q = q->next;
}
return true;
}
```
相关问题
设计一个算法Equal(),在链串上实现判断两个串是否相等的功能
算法思路:
首先比较两个链串的长度是否相等,若不相等,则两个串一定不相等;若相等,则逐个比较两个链串中的每个字符是否相等,若有不相等的字符,则两个串不相等,否则两个串相等。
算法步骤:
1. 定义一个函数Equal(str1, str2),其中str1和str2分别表示两个链串的头节点指针;
2. 定义两个指针p1和p2,分别指向str1和str2;
3. 定义两个变量len1和len2,分别表示str1和str2的长度;
4. 如果len1不等于len2,则返回false;
5. 从头节点开始,逐个比较p1和p2指向的字符是否相等,如果有不相等的字符,则返回false;
6. 如果比较完所有字符都相等,则返回true。
算法实现:
```
bool Equal(LinkStrNode *str1, LinkStrNode *str2) {
LinkStrNode *p1 = str1, *p2 = str2;
int len1 = 0, len2 = 0;
while (p1->next != NULL) {
len1++;
p1 = p1->next;
}
while (p2->next != NULL) {
len2++;
p2 = p2->next;
}
if (len1 != len2) {
return false;
}
p1 = str1->next;
p2 = str2->next;
while (p1 != NULL && p2 != NULL) {
if (p1->data != p2->data) {
return false;
}
p1 = p1->next;
p2 = p2->next;
}
return true;
}
```
字符串中判断两个字符串是否相等
要判断两个字符串是否相等,可以使用字符串比较操作符(==)或者字符串的比较方法(equals())。
使用字符串比较操作符(==):
```java
String str1 = "Hello";
String str2 = "Hello";
if (str1 == str2) {
System.out.println("两个字符串相等");
} else {
System.out.println("两个字符串不相等");
}
```
使用字符串的比较方法(equals()):
```java
String str1 = "Hello";
String str2 = "Hello";
if (str1.equals(str2)) {
System.out.println("两个字符串相等");
} else {
System.out.println("两个字符串不相等");
}
```
这两种方法都可以用来判断两个字符串是否相等,但是需要注意的是,使用比较操作符(==)比较的是两个字符串的引用是否相同,而使用比较方法(equals())比较的是两个字符串的内容是否相同。在一般情况下,推荐使用比较方法(equals())来判断两个字符串是否相等。
相关推荐
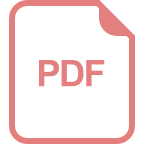
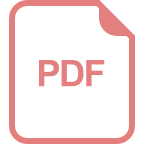
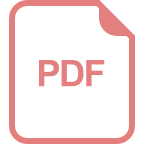












