现有动物类Animal,包含名称name和年龄age数据成员,返回年龄GetAge、显示信息Showhe。由动物类派生出羊类Sheep和驼类Camel,前者增加重量、后者增加品种两个数据成员,都有显示信息的函数。羊驼类Alpace由羊类Sheep和驼类Camel公派生而来,定义各个类并测试相关功能。
时间: 2024-02-11 17:03:54 浏览: 53
好的,这是一个关于面向对象编程的问题。根据您的描述,我可以为您提供以下代码示例:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Animal {
protected:
string name;
int age;
public:
Animal(string n, int a) : name(n), age(a) {}
int GetAge() { return age; }
void Show() {
cout << "Name: " << name << endl;
cout << "Age: " << age << endl;
}
};
class Sheep : public Animal {
private:
double weight;
public:
Sheep(string n, int a, double w) : Animal(n, a), weight(w) {}
void Show() {
Animal::Show();
cout << "Weight: " << weight << endl;
}
};
class Camel : public Animal {
private:
string breed;
public:
Camel(string n, int a, string b) : Animal(n, a), breed(b) {}
void Show() {
Animal::Show();
cout << "Breed: " << breed << endl;
}
};
class Alpaca : public Sheep, public Camel {
public:
Alpaca(string n, int a, double w, string b) : Sheep(n, a, w), Camel(n, a, b) {}
void Show() {
Sheep::Show();
Camel::Show();
}
};
int main() {
Sheep s("Dolly", 2, 50.5);
Camel c("Humphrey", 4, "Bactrian");
Alpaca a("Paco", 1, 30.2, "Dromedary");
s.Show();
cout << endl;
c.Show();
cout << endl;
a.Show();
return 0;
}
```
以上代码定义了 Animal、Sheep、Camel 和 Alpaca 四个类,分别表示动物、羊、驼和羊驼。其中 Animal 类包含名称和年龄两个数据成员,GetAge() 函数用于返回年龄,Show() 函数用于显示信息。Sheep 类和 Camel 类分别继承自 Animal 类,增加了重量和品种两个数据成员,同时重写了 Show() 函数以显示新增的数据成员。Alpaca 类继承自 Sheep 类和 Camel 类,因此具有两个父类的数据成员和函数成员。在主函数中,我们实例化了三个对象,分别为一个羊、一个驼和一个羊驼,然后分别调用它们的 Show() 函数以显示信息。
值得注意的是,由于 Alpaca 类同时继承自 Sheep 类和 Camel 类,因此在 Alpaca 类中需要使用虚继承(virtual inheritance)来解决菱形继承问题。但是由于本例中 Alpaca 类没有使用到 Animal 类的数据成员和函数成员,因此可以不需要将 Animal 类设置为虚基类。
阅读全文
相关推荐
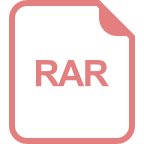
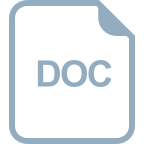

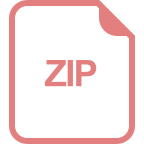
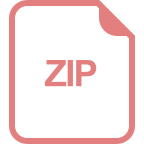
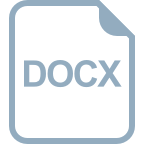
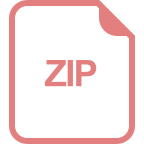
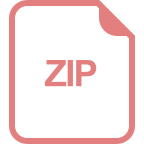
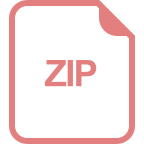
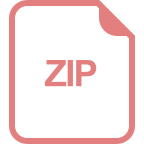
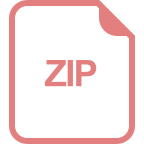
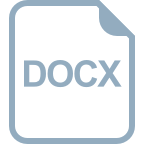