创建一个简易的前馈神经网络
时间: 2023-10-19 08:20:08 浏览: 33
好的,以下是一个简单的前馈神经网络的示例代码:
```
import numpy as np
class FeedforwardNeuralNetwork:
def __init__(self, input_size, output_size, hidden_layers=[10, 10], activation_function='sigmoid'):
self.input_size = input_size
self.output_size = output_size
self.hidden_layers = hidden_layers
self.activation_function = activation_function
# Initialize weights and biases for hidden layers
self.weights = []
self.biases = []
input_layer_size = input_size
for layer_size in hidden_layers:
weight_matrix = np.random.randn(layer_size, input_layer_size)
bias_vector = np.random.randn(layer_size, 1)
self.weights.append(weight_matrix)
self.biases.append(bias_vector)
input_layer_size = layer_size
# Initialize weights and biases for output layer
weight_matrix = np.random.randn(output_size, input_layer_size)
bias_vector = np.random.randn(output_size, 1)
self.weights.append(weight_matrix)
self.biases.append(bias_vector)
def sigmoid(self, z):
return 1 / (1 + np.exp(-z))
def relu(self, z):
return np.maximum(0, z)
def forward(self, X):
A = X
for i in range(len(self.weights)):
Z = np.dot(self.weights[i], A) + self.biases[i]
if self.activation_function == 'sigmoid':
A = self.sigmoid(Z)
elif self.activation_function == 'relu':
A = self.relu(Z)
return A
```
以上代码定义了一个类 `FeedforwardNeuralNetwork`,它接受输入大小、输出大小和隐藏层大小的参数,并且可以指定使用 `sigmoid` 或 `ReLU` 作为激活函数。通过 `weights` 和 `biases` 属性存储所有隐藏层和输出层的权重和偏置。`sigmoid` 和 `relu` 方法分别实现了 `sigmoid` 和 `ReLU` 激活函数。`forward` 方法接受输入 `X` 并计算网络输出。在每个隐藏层和输出层中,我们首先计算加权输入 `Z`,然后应用激活函数 `A`。最后,返回输出层的值。
你可以创建一个实例并使用 `forward` 方法计算输出,例如:
```
# Create a neural network with 2 input neurons, 1 output neuron, and 1 hidden layer with 3 neurons
network = FeedforwardNeuralNetwork(2, 1, [3])
# Compute the output for an input of [1, 2]
output = network.forward(np.array([1, 2]).reshape(-1, 1))
print(output)
```
输出将是一个表示网络预测的单个值。请注意,这个神经网络是非常简单的,并且仅用于演示目的。在实际应用中,你需要考虑更复杂的结构、更多的层和更多的神经元。同时,还需要进行更复杂的训练和调优过程来获得预期的结果。
相关推荐
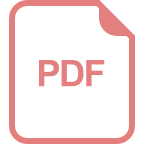

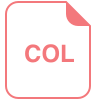


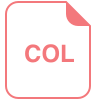









