帮我写一个前馈神经网络
时间: 2023-11-04 18:04:37 浏览: 38
首先需要确定神经网络的结构,包括输入层、隐藏层和输出层的节点数和激活函数。
假设我们要构建一个前馈神经网络,用于分类手写数字图像。我们将使用MNIST数据集,该数据集包含手写数字的图像和对应的标签。
输入层:MNIST数据集中的每个图像都是28x28像素的灰度图像,因此我们将输入层的节点数设置为784(28x28)。每个像素值将作为输入层的一个节点。
隐藏层:我们选择使用两个隐藏层,每个隐藏层包含64个节点。这些节点将使用ReLU激活函数。
输出层:由于MNIST数据集中有10个数字类别(0-9),我们将输出层的节点数设置为10。输出层使用softmax激活函数,用于将输出转换为概率分布。
接下来,我们需要确定网络的权重和偏差。我们可以使用随机初始化的权重和偏差。
然后,我们可以开始训练网络。我们将使用交叉熵损失函数,并使用反向传播算法来更新权重和偏差。我们还将使用随机梯度下降优化算法来加速训练过程。
这里是一个示例代码,用于实现一个前馈神经网络:
```
import tensorflow as tf
from tensorflow import keras
# Load the MNIST dataset
mnist = keras.datasets.mnist
(train_images, train_labels), (test_images, test_labels) = mnist.load_data()
# Normalize the pixel values to be between 0 and 1
train_images = train_images / 255.0
test_images = test_images / 255.0
# Define the neural network model
model = keras.Sequential([
keras.layers.Flatten(input_shape=(28, 28)),
keras.layers.Dense(64, activation='relu'),
keras.layers.Dense(64, activation='relu'),
keras.layers.Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='sgd',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Train the model
model.fit(train_images, train_labels, epochs=10, batch_size=32)
# Evaluate the model on the test dataset
test_loss, test_acc = model.evaluate(test_images, test_labels)
print('Test accuracy:', test_acc)
```
在这个代码片段中,我们首先加载MNIST数据集,然后对像素值进行归一化处理。接下来,我们定义了一个包含两个隐藏层的神经网络模型,并使用sgd优化器和交叉熵损失函数进行编译。我们使用fit()方法对模型进行训练,并在测试数据集上评估模型的准确性。
相关推荐
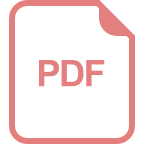
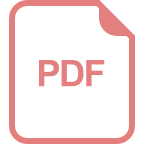
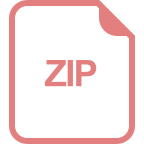














