1. Write a LC-3 subroutine for printing “Hello” for N times. Write a main program to input N from the keyboard, call the subroutine and then output “Hello” for N times.
时间: 2023-11-22 14:57:02 浏览: 131
Here's an example LC-3 subroutine and main program that will print "Hello" for N times:
```
; Subroutine to print "Hello" for N times
;
; Input: R0 contains the number of times to print "Hello"
; Output: None
.ORIG x3000
PRINT_LOOP:
LD R1, HELLO_STRING ; Load address of "Hello" string into R1
LD R2, ASCII_NEWLINE ; Load newline character into R2
ADD R0, R0, #-1 ; Decrement N
BRz PRINT_EXIT ; If N = 0, exit the loop
PUTS ; Print "Hello"
ADD R0, R0, #-1 ; Decrement N again
BRz PRINT_EXIT ; If N = 0, exit the loop
OUT ; Print newline
BRnzp PRINT_LOOP ; Loop back to start
PRINT_EXIT:
RET ; Return from subroutine
; Data section
;
; Define the "Hello" string and newline character in ASCII
HELLO_STRING .STRINGZ "Hello"
ASCII_NEWLINE .FILL x0A
.END
; Main program to input N and call the subroutine
;
; Input: None
; Output: None
.ORIG x4000
MAIN:
LEA R0, N ; Load address of N into R0
IN ; Read N from keyboard
JSR PRINT_HELLO ; Call subroutine to print "Hello" for N times
HALT ; Halt the program
; Subroutine to print "Hello" for N times
;
; Input: R0 contains the number of times to print "Hello"
; Output: None
PRINT_HELLO:
ST R0, SAVE_R0 ; Save R0 on the stack
JSR PRINT_LOOP ; Call the "Hello" printing loop
LD R0, SAVE_R0 ; Restore R0 from the stack
RET ; Return from subroutine
; Data section
;
; Define the input variable N and a temporary storage location
N .BLKW 1
SAVE_R0 .BLKW 1
.END
```
To run this program:
1. Assemble the code using LC-3 assembler.
2. Load the resulting .obj file into the LC-3 simulator.
3. Run the program and enter a value for N when prompted.
4. The program will print "Hello" for N times.
阅读全文
相关推荐
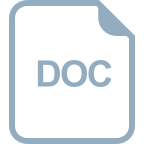
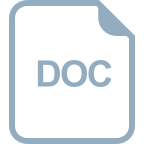
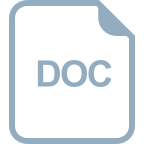

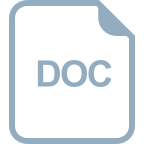
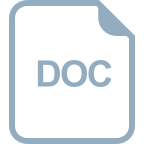
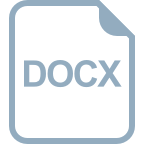
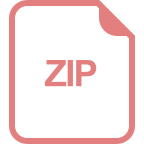
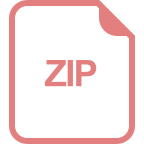