用Java的NIO技术写一个Knock Knock游戏的客户机/服务器代码
时间: 2024-02-17 08:01:33 浏览: 32
好的,我可以为您提供一个基本的Knock Knock游戏的客户机/服务器代码。此代码使用Java NIO技术来处理客户端和服务器端之间的通信。
这是服务器端的代码:
```java
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.SelectionKey;
import java.nio.channels.Selector;
import java.nio.channels.ServerSocketChannel;
import java.nio.channels.SocketChannel;
import java.util.Iterator;
public class KnockKnockServer {
private static final int BUFFER_SIZE = 1024;
private static final int TIMEOUT = 3000;
public static void main(String[] args) throws IOException {
int port = 8080;
if (args.length > 0) {
port = Integer.parseInt(args[0]);
}
Selector selector = Selector.open();
ServerSocketChannel serverSocketChannel = ServerSocketChannel.open();
serverSocketChannel.socket().bind(new InetSocketAddress(port));
serverSocketChannel.configureBlocking(false);
serverSocketChannel.register(selector, SelectionKey.OP_ACCEPT);
while (true) {
if (selector.select(TIMEOUT) == 0) {
continue;
}
Iterator<SelectionKey> iterator = selector.selectedKeys().iterator();
while (iterator.hasNext()) {
SelectionKey key = iterator.next();
if (key.isAcceptable()) {
handleAccept(key, selector);
}
if (key.isReadable()) {
handleRead(key, selector);
}
iterator.remove();
}
}
}
private static void handleAccept(SelectionKey key, Selector selector) throws IOException {
ServerSocketChannel serverSocketChannel = (ServerSocketChannel) key.channel();
SocketChannel socketChannel = serverSocketChannel.accept();
socketChannel.configureBlocking(false);
socketChannel.register(selector, SelectionKey.OP_READ, ByteBuffer.allocate(BUFFER_SIZE));
}
private static void handleRead(SelectionKey key, Selector selector) throws IOException {
SocketChannel socketChannel = (SocketChannel) key.channel();
ByteBuffer buffer = (ByteBuffer) key.attachment();
int bytesRead = socketChannel.read(buffer);
if (bytesRead == -1) {
socketChannel.close();
return;
}
if (bytesRead > 0) {
String message = new String(buffer.array()).trim();
if (message.equalsIgnoreCase("Knock Knock")) {
buffer.clear();
buffer.put("Who's there?".getBytes());
buffer.flip();
socketChannel.write(buffer);
} else if (message.equalsIgnoreCase("Boo")) {
buffer.clear();
buffer.put("Don't cry, it's only a joke.".getBytes());
buffer.flip();
socketChannel.write(buffer);
} else {
buffer.clear();
buffer.put("You're supposed to say \"Knock Knock\"!".getBytes());
buffer.flip();
socketChannel.write(buffer);
}
}
}
}
```
这是客户端的代码:
```java
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.SocketChannel;
import java.util.Scanner;
public class KnockKnockClient {
private static final int BUFFER_SIZE = 1024;
public static void main(String[] args) throws IOException {
String host = "localhost";
int port = 8080;
if (args.length > 0) {
host = args[0];
}
if (args.length > 1) {
port = Integer.parseInt(args[1]);
}
InetSocketAddress serverAddress = new InetSocketAddress(host, port);
SocketChannel socketChannel = SocketChannel.open(serverAddress);
socketChannel.configureBlocking(false);
ByteBuffer buffer = ByteBuffer.allocate(BUFFER_SIZE);
Scanner scanner = new Scanner(System.in);
while (true) {
String message = scanner.nextLine();
buffer.clear();
buffer.put(message.getBytes());
buffer.flip();
socketChannel.write(buffer);
buffer.clear();
int bytesRead = socketChannel.read(buffer);
if (bytesRead == -1) {
socketChannel.close();
return;
}
if (bytesRead > 0) {
String response = new String(buffer.array()).trim();
System.out.println(response);
}
}
}
}
```
运行服务器端代码后,可以在另一个终端中运行客户端代码。在客户端中输入“Knock Knock”,服务器将响应“Who's there?”。然后,在客户端中输入“Boo”,服务器将响应“Don't cry, it's only a joke.”。如果客户端输入其他内容,则服务器将响应“You're supposed to say \"Knock Knock\"!”。
相关推荐
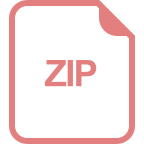
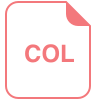
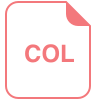
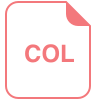
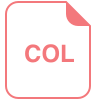
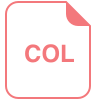









